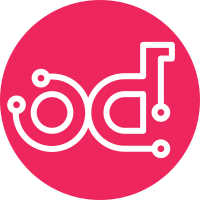
It turns out to be a giant PITA to do keystone v3 auth by hand, as the bmc install script was trying to. Different clouds require a different combination of auth values set, and os-client-config already exists to handle these variations. To do this, the os-client-config data is passed directly into the bmc install script as json, which is then written to clouds.yaml so it can be used by the clients. In theory this should mean that the bmc can handle any cloud where we were able to deploy the heat stack because it's using the exact same auth data. In addition, this change makes os-client-config mandatory on the bmc image. This is not a meaningful change though because it was already pulled in by the clients, so it should already exist on any bmc images in the wild. It also deprecates the old method of providing auth data to the bmc. For the moment it should continue to work, but the os-client-config method should be used going forward. Note that by default the bmc will now read auth parameters from the environment, which is actually an improvement over how it worked before when running interactively.
71 lines
2.6 KiB
Python
71 lines
2.6 KiB
Python
# Copyright 2017 Red Hat Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import json
|
|
import os
|
|
import sys
|
|
|
|
from keystoneclient.v2_0 import client as keystone_client
|
|
from keystoneclient.v3 import client as keystone_v3_client
|
|
import os_client_config
|
|
|
|
|
|
# Older versions of os-client-config pop this from the environment when
|
|
# make_client is called. Cache it on import so we know what the original
|
|
# value was, regardless of any funny business that happens later.
|
|
OS_CLOUD = os.environ.get('OS_CLOUD')
|
|
|
|
|
|
def _create_auth_parameters():
|
|
"""Read keystone auth parameters from appropriate source
|
|
|
|
If the environment variable OS_CLOUD is set, read the auth information
|
|
from os_client_config. Otherwise, read it from environment variables.
|
|
When reading from the environment, also validate that all of the required
|
|
values are set.
|
|
|
|
:returns: A dict containing the following keys: os_user, os_password,
|
|
os_tenant, os_auth_url, os_project, os_user_domain,
|
|
os_project_domain.
|
|
"""
|
|
config = os_client_config.OpenStackConfig().get_one_cloud(OS_CLOUD)
|
|
auth = config.config['auth']
|
|
username = auth['username']
|
|
password = auth['password']
|
|
# os_client_config seems to always call this project_name
|
|
tenant = auth['project_name']
|
|
auth_url = auth['auth_url']
|
|
project = auth['project_name']
|
|
user_domain = (auth.get('user_domain_name') or
|
|
auth.get('user_domain_id', ''))
|
|
project_domain = (auth.get('project_domain_name') or
|
|
auth.get('project_domain_id', ''))
|
|
|
|
return {'os_user': username,
|
|
'os_password': password,
|
|
'os_tenant': tenant,
|
|
'os_auth_url': auth_url,
|
|
'os_project': project,
|
|
'os_user_domain': user_domain,
|
|
'os_project_domain': project_domain,
|
|
}
|
|
|
|
def _cloud_json():
|
|
"""Return the current cloud's data in JSON
|
|
|
|
Retrieves the cloud from os-client-config and serializes it to JSON.
|
|
"""
|
|
config = os_client_config.OpenStackConfig().get_one_cloud(OS_CLOUD)
|
|
return json.dumps(config.config)
|