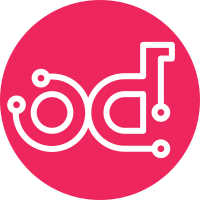
Instead of just tracking projects that are part of the integrated gate lets start tracking all of openstack. Defining openstack as everything in openstack/ openstack-infra/ and openstack-dev/ As we move away from a single integrated gate, the list of what should be in this repo under the old definition is quickly dropping. Instead of using this to just help debug integrated gate failures, we can use this to better track all openstack activity. Knowing the basic nature of a gate failure means you can quickly narrow down the number of repos that can possibly trigger the failure. That combined with ElasticSearch data to identify a window of time the issue was introduced during makes it easy to quickly narrow the number of patches that could have possibly cause the problems. Change-Id: I9c04a4d53f4628e0419d744b7e047ad75c0d133d
64 lines
1.8 KiB
Python
Executable File
64 lines
1.8 KiB
Python
Executable File
#!/usr/bin/python
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import json
|
|
|
|
import requests
|
|
|
|
TEMPLATE = """[submodule "%s"]
|
|
\tpath = %s
|
|
\turl = https://review.openstack.org/%s.git
|
|
\tbranch = .
|
|
"""
|
|
|
|
# only return projects starting with openstack
|
|
CONFIG = ("https://review.openstack.org:443/projects/?p=openstack")
|
|
|
|
|
|
def find_integrated_gate_projects():
|
|
r = requests.get(CONFIG)
|
|
# strip off first few chars because 'the JSON response body starts with a
|
|
# magic prefix line that must be stripped before feeding the rest of the
|
|
# response body to a JSON parser'
|
|
# https://review.openstack.org/Documentation/rest-api.html
|
|
gerrit_projects = json.loads(r.text[4:])
|
|
|
|
# Ignore openstack-attic
|
|
orgs = ['openstack/', 'openstack-infra/', 'openstack-dev/']
|
|
blacklist = ['openstack/openstack']
|
|
|
|
projects = []
|
|
for project in gerrit_projects:
|
|
if any(project.startswith(org) for org in orgs):
|
|
if project not in blacklist:
|
|
projects.append(project)
|
|
return projects
|
|
|
|
|
|
def gen_gitmodules(projects):
|
|
projects = sorted(projects)
|
|
with open(".gitmodules", 'w') as f:
|
|
for p in projects:
|
|
ns, name = p.split('/')
|
|
f.write(TEMPLATE % (name, name, p))
|
|
|
|
|
|
def main():
|
|
projects = find_integrated_gate_projects()
|
|
gen_gitmodules(projects)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
main()
|