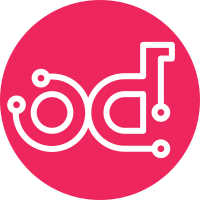
Document in which version new types and functions were added using ".. versionadded:: x.y". Document changes using ".. versionchanged:: x.y." For watchdog module, add the versionadded tag in the module top docstring, not on each type/function. Add docstring to ssh_execute(). I used "git blame" + "git tag --contains=SHA1" to find these version, and then I checked manually each version. Change-Id: I56a7d8a4335833e9b49eedab026d20adfeedf942
79 lines
2.9 KiB
Python
79 lines
2.9 KiB
Python
# Copyright 2011 OpenStack Foundation.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import fixtures
|
|
from oslo_config import fixture as config
|
|
|
|
from oslo_concurrency import lockutils
|
|
|
|
|
|
class LockFixture(fixtures.Fixture):
|
|
"""External locking fixture.
|
|
|
|
This fixture is basically an alternative to the synchronized decorator with
|
|
the external flag so that tearDowns and addCleanups will be included in
|
|
the lock context for locking between tests. The fixture is recommended to
|
|
be the first line in a test method, like so::
|
|
|
|
def test_method(self):
|
|
self.useFixture(LockFixture('lock_name'))
|
|
...
|
|
|
|
or the first line in setUp if all the test methods in the class are
|
|
required to be serialized. Something like::
|
|
|
|
class TestCase(testtools.testcase):
|
|
def setUp(self):
|
|
self.useFixture(LockFixture('lock_name'))
|
|
super(TestCase, self).setUp()
|
|
...
|
|
|
|
This is because addCleanups are put on a LIFO queue that gets run after the
|
|
test method exits. (either by completing or raising an exception)
|
|
"""
|
|
def __init__(self, name, lock_file_prefix=None):
|
|
self.mgr = lockutils.lock(name, lock_file_prefix, True)
|
|
|
|
def setUp(self):
|
|
super(LockFixture, self).setUp()
|
|
self.addCleanup(self.mgr.__exit__, None, None, None)
|
|
self.lock = self.mgr.__enter__()
|
|
|
|
|
|
class ExternalLockFixture(fixtures.Fixture):
|
|
"""Configure lock_path so external locks can be used in unit tests.
|
|
|
|
Creates a temporary directory to hold file locks and sets the oslo.config
|
|
lock_path opt to use it. This can be used to enable external locking
|
|
on a per-test basis, rather than globally with the OSLO_LOCK_PATH
|
|
environment variable.
|
|
|
|
Example::
|
|
|
|
def test_method(self):
|
|
self.useFixture(ExternalLockFixture())
|
|
something_that_needs_external_locks()
|
|
|
|
Alternatively, the useFixture call could be placed in a test class's
|
|
setUp method to provide this functionality to all tests in the class.
|
|
|
|
.. versionadded:: 0.3
|
|
"""
|
|
def setUp(self):
|
|
super(ExternalLockFixture, self).setUp()
|
|
temp_dir = self.useFixture(fixtures.TempDir())
|
|
conf = self.useFixture(config.Config(lockutils.CONF)).config
|
|
conf(lock_path=temp_dir.path, group='oslo_concurrency')
|