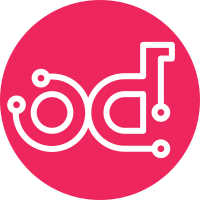
The main issue with eventlet.green.zmq is that libzmq as a C-library is completely monkey-patch unfriendly. So any blocking call inside the native library makes calling process stuck. We can't avoid this actually in an absolutely normal situation when a client appears earlier than listener we have all client process get stuck until listener raised. If the listener for example is also blocked awaiting for some other service to appear we have a chain of locks which may occasionally result in a dead-lock. The other situation with Notifier is quite similar. For that reason zmq-broker was restored, but now it serves as an outgoing queue on a client side. Servers remained the same dynamically port-binded. Now all clients can still use green-zmq, but presence of the broker-queue on a host guarantees that green threads will never blocked in a client because all messages will wait their listeners inside the broker queue. The broker process's modules are not monkey-patched, they make use of native threading and native zmq. Possibility to run without broker also remains. The option zmq_use_broker introduced for that reason. Closes-Bug: #1497315 Change-Id: I786b100fd6ee1cf4b99139db0ca044d358d36345
43 lines
1.3 KiB
Python
43 lines
1.3 KiB
Python
# Copyright 2015 Mirantis, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import contextlib
|
|
import logging
|
|
import sys
|
|
|
|
from oslo_config import cfg
|
|
|
|
from oslo_messaging._drivers import impl_zmq
|
|
from oslo_messaging._drivers.zmq_driver.broker import zmq_broker
|
|
from oslo_messaging._executors import impl_pooledexecutor
|
|
|
|
CONF = cfg.CONF
|
|
CONF.register_opts(impl_zmq.zmq_opts)
|
|
CONF.register_opts(impl_pooledexecutor._pool_opts)
|
|
# TODO(ozamiatin): Move this option assignment to an external config file
|
|
# Use efficient zmq poller in real-world deployment
|
|
CONF.rpc_zmq_native = True
|
|
|
|
|
|
def main():
|
|
CONF(sys.argv[1:], project='oslo')
|
|
logging.basicConfig(level=logging.DEBUG)
|
|
|
|
with contextlib.closing(zmq_broker.ZmqBroker(CONF)) as reactor:
|
|
reactor.start()
|
|
reactor.wait()
|
|
|
|
if __name__ == "__main__":
|
|
main()
|