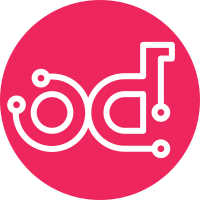
This makes the RPC version support three elements, adding a "revision" in addition to the major and minor version. The revision would always be zero for the master branch of a service, but could be incremented for stable versions. This provides us some room to backport fixes that affect RPC versions in such a way that would avoid breaking the version lineage for systems running stable versions that may some day be involved in a rolling upgrade to a version from master. I didn't find any tests for version_is_compatible(), so I added some for existing version scenarios, as well as new ones with revisions. They also serve to validate that this doesn't break anything for code using two-element versions (the expectation is that two-element versions will still be used everywhere until a third is needed). Porting chages from Change-Id I239c17a3e305f572493498c4b96ee3c7514c5881 to oslo-incubator Change-Id: I4fa7b0be14a7afba36136a746b76036355f119b2
50 lines
2.0 KiB
Python
50 lines
2.0 KiB
Python
|
|
# Copyright 2013 Red Hat, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from oslo.messaging import _utils as utils
|
|
from tests import utils as test_utils
|
|
|
|
|
|
class PoolTestCase(test_utils.BaseTestCase):
|
|
def test_version_is_compatible_same(self):
|
|
self.assertTrue(utils.version_is_compatible('1.23', '1.23'))
|
|
|
|
def test_version_is_compatible_newer_minor(self):
|
|
self.assertTrue(utils.version_is_compatible('1.24', '1.23'))
|
|
|
|
def test_version_is_compatible_older_minor(self):
|
|
self.assertFalse(utils.version_is_compatible('1.22', '1.23'))
|
|
|
|
def test_version_is_compatible_major_difference1(self):
|
|
self.assertFalse(utils.version_is_compatible('2.23', '1.23'))
|
|
|
|
def test_version_is_compatible_major_difference2(self):
|
|
self.assertFalse(utils.version_is_compatible('1.23', '2.23'))
|
|
|
|
def test_version_is_compatible_newer_rev(self):
|
|
self.assertFalse(utils.version_is_compatible('1.23', '1.23.1'))
|
|
|
|
def test_version_is_compatible_newer_rev_both(self):
|
|
self.assertFalse(utils.version_is_compatible('1.23.1', '1.23.2'))
|
|
|
|
def test_version_is_compatible_older_rev_both(self):
|
|
self.assertTrue(utils.version_is_compatible('1.23.2', '1.23.1'))
|
|
|
|
def test_version_is_compatible_older_rev(self):
|
|
self.assertTrue(utils.version_is_compatible('1.24', '1.23.1'))
|
|
|
|
def test_version_is_compatible_no_rev_is_zero(self):
|
|
self.assertTrue(utils.version_is_compatible('1.23.0', '1.23'))
|