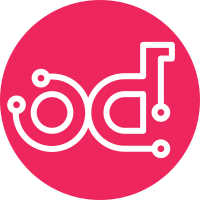
In previous patch we changed behavior or profiler lib. Currently profiler want create any notification if hmac key is not setted up and all messages are not properly signed by it. So to avoid mistakes (e.g. forgot to init profiler with hmac key) this argument should be required (not optional) As well a lot of improvments in docs related to profiler module Change-Id: I74e05234a5638ffd93dbac9180d4497fcb5093ac
146 lines
4.7 KiB
Python
146 lines
4.7 KiB
Python
# Copyright 2014 Mirantis Inc.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import collections
|
|
import mock
|
|
|
|
from osprofiler import profiler
|
|
|
|
from tests import test
|
|
|
|
|
|
class ProfilerGlobMethodsTestCase(test.TestCase):
|
|
|
|
def test_get_profiler_not_inited(self):
|
|
profiler._clean()
|
|
self.assertIsNone(profiler.get_profiler())
|
|
|
|
def test_get_profiler_and_init(self):
|
|
p = profiler.init("secret", base_id="1", parent_id="2")
|
|
self.assertEqual(profiler.get_profiler(), p)
|
|
|
|
self.assertEqual(p.get_base_id(), "1")
|
|
# NOTE(boris-42): until we make first start we don't have
|
|
self.assertEqual(p.get_id(), "2")
|
|
|
|
def test_start_not_inited(self):
|
|
profiler._clean()
|
|
profiler.start("name")
|
|
|
|
def test_start(self):
|
|
p = profiler.init("secret", base_id="1", parent_id="2")
|
|
p.start = mock.MagicMock()
|
|
profiler.start("name", info="info")
|
|
p.start.assert_called_once_with("name", info="info")
|
|
|
|
def test_stop_not_inited(self):
|
|
profiler._clean()
|
|
profiler.stop()
|
|
|
|
def test_stop(self):
|
|
p = profiler.init("secret", base_id="1", parent_id="2")
|
|
p.stop = mock.MagicMock()
|
|
profiler.stop(info="info")
|
|
p.stop.assert_called_once_with(info="info")
|
|
|
|
|
|
class ProfilerTestCase(test.TestCase):
|
|
|
|
def test_profiler_get_base_id(self):
|
|
prof = profiler.Profiler("secret", base_id="1", parent_id="2")
|
|
self.assertEqual(prof.get_base_id(), "1")
|
|
|
|
@mock.patch("osprofiler.profiler.uuid.uuid4")
|
|
def test_profiler_get_parent_id(self, mock_uuid4):
|
|
mock_uuid4.return_value = "42"
|
|
prof = profiler.Profiler("secret", base_id="1", parent_id="2")
|
|
prof.start("test")
|
|
self.assertEqual(prof.get_parent_id(), "2")
|
|
|
|
@mock.patch("osprofiler.profiler.uuid.uuid4")
|
|
def test_profiler_get_base_id_unset_case(self, mock_uuid4):
|
|
mock_uuid4.return_value = "42"
|
|
prof = profiler.Profiler("secret")
|
|
self.assertEqual(prof.get_base_id(), "42")
|
|
self.assertEqual(prof.get_parent_id(), "42")
|
|
|
|
@mock.patch("osprofiler.profiler.uuid.uuid4")
|
|
def test_profiler_get_id(self, mock_uuid4):
|
|
mock_uuid4.return_value = "43"
|
|
prof = profiler.Profiler("secret")
|
|
prof.start("test")
|
|
self.assertEqual(prof.get_id(), "43")
|
|
|
|
@mock.patch("osprofiler.profiler.uuid.uuid4")
|
|
@mock.patch("osprofiler.profiler.notifier.notify")
|
|
def test_profiler_start(self, mock_notify, mock_uuid4):
|
|
mock_uuid4.return_value = "44"
|
|
|
|
info = {"some": "info"}
|
|
payload = {
|
|
"name": "test-start",
|
|
"base_id": "1",
|
|
"parent_id": "2",
|
|
"trace_id": "44",
|
|
"info": info
|
|
}
|
|
|
|
prof = profiler.Profiler("secret", base_id="1", parent_id="2")
|
|
prof.start("test", info=info)
|
|
|
|
mock_notify.assert_called_once_with(payload)
|
|
|
|
@mock.patch("osprofiler.profiler.notifier.notify")
|
|
def test_profiler_stop(self, mock_notify):
|
|
prof = profiler.Profiler("secret", base_id="1", parent_id="2")
|
|
prof._trace_stack.append("44")
|
|
prof._name.append("abc")
|
|
|
|
info = {"some": "info"}
|
|
prof.stop(info=info)
|
|
|
|
payload = {
|
|
"name": "abc-stop",
|
|
"base_id": "1",
|
|
"parent_id": "2",
|
|
"trace_id": "44",
|
|
"info": info
|
|
}
|
|
|
|
mock_notify.assert_called_once_with(payload)
|
|
self.assertEqual(len(prof._name), 0)
|
|
self.assertEqual(prof._trace_stack, collections.deque(["1", "2"]))
|
|
|
|
def test_profiler_hmac(self):
|
|
hmac = "secret"
|
|
prof = profiler.Profiler(hmac, base_id="1", parent_id="2")
|
|
self.assertEqual(hmac, prof.hmac_key)
|
|
|
|
|
|
class TraceTestCase(test.TestCase):
|
|
|
|
@mock.patch("osprofiler.profiler.stop")
|
|
@mock.patch("osprofiler.profiler.start")
|
|
def test_trace(self, mock_start, mock_stop):
|
|
|
|
with profiler.Trace("a", info="a1"):
|
|
mock_start.assert_called_once_with("a", info="a1")
|
|
mock_start.reset_mock()
|
|
with profiler.Trace("b", info="b1"):
|
|
mock_start.assert_called_once_with("b", info="b1")
|
|
mock_stop.assert_called_once_with()
|
|
mock_stop.reset_mock()
|
|
mock_stop.assert_called_once_with()
|