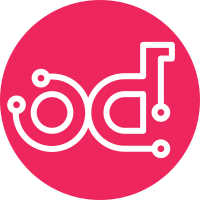
When Load Balancer and its member has FIP assigned and environment is configured to use DVR the member FIP needs to be centralized. It is current core OVN limitation, that should be solved in [1]. This patch adds this mechanism to OVN Client and OVN Octavia provider driver. It covers cases: 1) FIP association on port that is a member of some LB - make it centralized. 2) FIP association on LB VIP - find a members FIPs and centralized them. 3) Add a member to LB that has FIP already configured - checks if a member has FIP and centralize it. 4) The reverse of each of the above cases. In addition I needed to extend OVN LB member external_id entry to add an information about member subnet_id in order to easly track member port from mechanism OVN driver. That means I needed also to support both old and new conventions. This patch adds also this code. Old convention: member_`member_id`_`ip_address`:`port` New convention: member_`member_id`_`ip_address`:`port`_`subnet_id` [1] https://bugzilla.redhat.com/show_bug.cgi?id=1793897 Related-Bug: #1860662 Change-Id: I254f0ac28f7585b699a8238e01ffb37dd70282ef (cherry picked from networking-ovn commit 57ac38921efa6bbf0bc4a22950355256cc3ebe6d)
52 lines
2.0 KiB
Python
52 lines
2.0 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from oslo_utils import netutils
|
|
|
|
from ovn_octavia_provider.common import constants
|
|
|
|
|
|
def ovn_name(id):
|
|
# The name of the OVN entry will be neutron-<UUID>
|
|
# This is due to the fact that the OVN application checks if the name
|
|
# is a UUID. If so then there will be no matches.
|
|
# We prefix the UUID to enable us to use the Neutron UUID when
|
|
# updating, deleting etc.
|
|
return 'neutron-%s' % id
|
|
|
|
|
|
def ovn_lrouter_port_name(id):
|
|
# The name of the OVN lrouter port entry will be lrp-<UUID>
|
|
# This is to distinguish with the name of the connected lswitch patch port,
|
|
# which is named with neutron port uuid, so that OVS patch ports are
|
|
# generated properly. The pairing patch port names will be:
|
|
# - patch-lrp-<UUID>-to-<UUID>
|
|
# - patch-<UUID>-to-lrp-<UUID>
|
|
# lrp stands for Logical Router Port
|
|
return constants.LRP_PREFIX + '%s' % id
|
|
|
|
|
|
def remove_macs_from_lsp_addresses(addresses):
|
|
"""Remove the mac addreses from the Logical_Switch_Port addresses column.
|
|
|
|
:param addresses: The list of addresses from the Logical_Switch_Port.
|
|
Example: ["80:fa:5b:06:72:b7 158.36.44.22",
|
|
"ff:ff:ff:ff:ff:ff 10.0.0.2"]
|
|
:returns: A list of IP addesses (v4 and v6)
|
|
"""
|
|
ip_list = []
|
|
for addr in addresses:
|
|
ip_list.extend([x for x in addr.split() if
|
|
(netutils.is_valid_ipv4(x) or
|
|
netutils.is_valid_ipv6(x))])
|
|
return ip_list
|