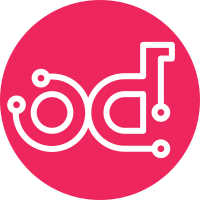
This script is unnecessarily complex for what it is doing, which is essentially pre-seeding the source-repositories cache for devstack. As we can see from I41e81d6bac98875eecde2376e0865784626e11a8 it's very confusing having large parts of the source-repositories script copy-pasted as a separate element and has led several of us down the wrong path. Strip this script back to the simple thing it is doing, which is checking out/updating the source-repositories devstack cache. I have tested this by building an image with a warm cache and with a cold cache, in both cases the checkout was found and the list of images to cache in 55-cache-devstack-repos was found. Change-Id: I6c686312de102cbe438585e26bf6986e06b6f41c
89 lines
3.2 KiB
Bash
Executable File
89 lines
3.2 KiB
Bash
Executable File
#!/bin/bash
|
|
|
|
# Copyright (c) 2012-2014 Hewlett-Packard Development Company, L.P.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
|
|
# implied.
|
|
#
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
# dib-lint: disable=setpipefail
|
|
if [ ${DIB_DEBUG_TRACE:-0} -gt 0 ]; then
|
|
set -x
|
|
fi
|
|
set -eu
|
|
|
|
# We need a copy of the devstack repo to query for the list of image
|
|
# files to cached by the source-repositories element (see
|
|
# 55-cache-devstack-repos)
|
|
#
|
|
# This script is a hack to use the source-repositories repo cache to
|
|
# get early access to the devstack checkout (rather than doing a whole
|
|
# separate clone of the devstack tree). In essence, this script knows
|
|
# the path of the devstack in the source-repositories cache and
|
|
# creates/updates it. To get the gist of where this comes from, see
|
|
# dib's
|
|
# elements/source-repositories/extra-data.d/98-source-repositories
|
|
#
|
|
# This could be removed if we could ever source and call the
|
|
# clone/update functions asynchronously from the source-repositories
|
|
# dib element.
|
|
|
|
function get_devstack_from_cache {
|
|
|
|
local REPONAME=devstack
|
|
local REPOTYPE=git
|
|
local REPOPATH=/opt/git/openstack-dev/devstack
|
|
local REPOLOCATION=git://git.openstack.org/openstack-dev/devstack.git
|
|
local REPOREF=master
|
|
|
|
local REPO_DEST=$TMP_MOUNT_PATH$REPOPATH
|
|
local REPO_SUB_DIRECTORY=$(dirname $REPO_DEST)
|
|
sudo mkdir -p $REPO_SUB_DIRECTORY
|
|
|
|
# build the same cache name as source-repositories
|
|
CACHE_NAME=$(echo "${REPOTYPE}_${REPOLOCATION}" | sha1sum | awk '{ print $1 }' )
|
|
CACHE_NAME=${REPONAME//[^A-Za-z0-9]/_}_${CACHE_NAME}
|
|
CACHE_PATH=${CACHE_BASE}/$CACHE_NAME
|
|
|
|
# clone repo if not in cache
|
|
if [ ! -e "$CACHE_PATH" ] ; then
|
|
echo "early-source-repo: Caching $REPONAME from $REPOLOCATION in $CACHE_PATH"
|
|
git clone $REPOLOCATION $CACHE_PATH.tmp
|
|
mv ${CACHE_PATH}{.tmp,}
|
|
fi
|
|
|
|
# update cached repo
|
|
if [ -z "$DIB_OFFLINE" ] ; then
|
|
echo "early-source-repo: Updating cache of $REPOLOCATION in $CACHE_PATH with ref $REPOREF"
|
|
# note, we could update *all* refs here (+refs/*:refs/* and
|
|
# create a really full mirror. but that brings in a lot of
|
|
# change refs from gerrit which traditionally we haven't used.
|
|
# Also, we only really care about refs/remotes/* as the
|
|
# devstack caching script goes through these branches to
|
|
# decide what to checkout. We keep refs/heads/* for
|
|
# historical reasons...)
|
|
git --git-dir=$CACHE_PATH/.git fetch --prune --update-head-ok \
|
|
$REPOLOCATION \
|
|
+refs/heads/*:refs/heads/* +refs/remotes/*:refs/remotes/*
|
|
fi
|
|
|
|
# clone the updated repo to our destination
|
|
sudo git clone $CACHE_PATH $REPO_DEST
|
|
}
|
|
|
|
# in case source-repositories hasn't run yet, make the cache
|
|
CACHE_BASE=$DIB_IMAGE_CACHE/source-repositories
|
|
mkdir -p $CACHE_BASE
|
|
|
|
get_devstack_from_cache
|