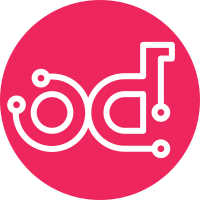
Change I2d1638296f264784333ba1745f75490d81b9ca00 overwrote the content flag and now the script does not return JSON as expected but XML. Change it to continue returning JSON. Also, use newer ProjectVersionResource REST API since the old one is deprecated. Change-Id: Idf493813d20d40cc92aaaffbe23db227eb1a213a
56 lines
1.9 KiB
Python
Executable File
56 lines
1.9 KiB
Python
Executable File
#!/usr/bin/env python
|
|
|
|
# Copyright (c) 2015 Hewlett-Packard Development Company, L.P.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import argparse
|
|
import os
|
|
import json
|
|
import sys
|
|
from ZanataUtils import IniConfig, ZanataRestService
|
|
|
|
|
|
def get_args():
|
|
parser = argparse.ArgumentParser(description='Check if a version for the '
|
|
'specified project exists on the Zanata '
|
|
'server')
|
|
parser.add_argument('-p', '--project', required=True)
|
|
parser.add_argument('-v', '--version', required=True)
|
|
parser.add_argument('--no-verify', action='store_false', dest='verify',
|
|
help='Do not perform HTTPS certificate verification')
|
|
return parser.parse_args()
|
|
|
|
|
|
def main():
|
|
args = get_args()
|
|
zc = IniConfig(os.path.expanduser('~/.config/zanata.ini'))
|
|
rest_service = ZanataRestService(zc, content_type='application/json',
|
|
accept='application/json',
|
|
verify=args.verify)
|
|
try:
|
|
r = rest_service.query(
|
|
'/rest/project/%s/version/%s'
|
|
% (args.project, args.version))
|
|
except ValueError:
|
|
sys.exit(1)
|
|
if r.status_code == 200:
|
|
details = json.loads(r.content)
|
|
if details['status'] == 'READONLY':
|
|
sys.exit(1)
|
|
sys.exit(0)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
main()
|