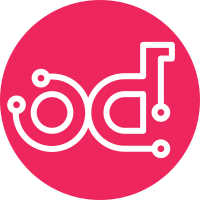
Add ironic api and inspector authtoken classes to configure keystone authtoken related parameters. Unit tests are updated accordingly. Deprecate some parameters in api and inspectore classes: - ironic::api|inspector::admin_tenant_name is deprecated in favor of ironic::api|inspector::authtoken::project_name. - ironic::api|inspector::admin_user is deprecated in favor of ironic::api|inspector::authtoken::username. - ironic::api|inspector::admin_password is deprecated in favor of ironic::api|inspector::authtoken::password. - ironic::api|inspector::identity_uri is deprecated in favor of ironic::api|inspector::authtoken::auth_uri. - ironic::api|inspector::auth_uri is deprecated in favor of ironic::api|inspector::authtoken::auth_uri. - ironic::api::memcached_servers is deprecated in favor of ironic::api::authtoken::memcached_servers. Closes-bug: #1604463 Change-Id: Idcb9557ab0b42b2a0dba7cf2ab6a5ccf52dd1d23
104 lines
2.9 KiB
Ruby
104 lines
2.9 KiB
Ruby
require 'puppet'
|
|
require 'spec_helper'
|
|
require 'puppet/provider/ironic'
|
|
require 'tempfile'
|
|
|
|
describe Puppet::Provider::Ironic do
|
|
|
|
def klass
|
|
described_class
|
|
end
|
|
|
|
let :credential_hash do
|
|
{
|
|
'project_name' => 'admin_tenant',
|
|
'username' => 'admin',
|
|
'password' => 'password',
|
|
'auth_uri' => 'https://192.168.56.210:35357/',
|
|
'project_domain_name' => 'admin_tenant_domain',
|
|
'user_domain_name' => 'admin_domain',
|
|
}
|
|
end
|
|
|
|
let :credential_error do
|
|
/Ironic types will not work/
|
|
end
|
|
|
|
after :each do
|
|
klass.reset
|
|
end
|
|
|
|
describe 'when determining credentials' do
|
|
|
|
it 'should fail if config is empty' do
|
|
conf = {}
|
|
klass.expects(:ironic_conf).returns(conf)
|
|
expect do
|
|
klass.ironic_credentials
|
|
end.to raise_error(Puppet::Error, credential_error)
|
|
end
|
|
|
|
it 'should fail if config does not have keystone_authtoken section.' do
|
|
conf = {'foo' => 'bar'}
|
|
klass.expects(:ironic_conf).returns(conf)
|
|
expect do
|
|
klass.ironic_credentials
|
|
end.to raise_error(Puppet::Error, credential_error)
|
|
end
|
|
|
|
it 'should fail if config does not contain all auth params' do
|
|
conf = {'keystone_authtoken' => {'invalid_value' => 'foo'}}
|
|
klass.expects(:ironic_conf).returns(conf)
|
|
expect do
|
|
klass.ironic_credentials
|
|
end.to raise_error(Puppet::Error, credential_error)
|
|
end
|
|
|
|
end
|
|
|
|
describe 'when invoking the ironic cli' do
|
|
|
|
it 'should set auth credentials in the environment' do
|
|
authenv = {
|
|
:OS_AUTH_URL => credential_hash['auth_uri'],
|
|
:OS_USERNAME => credential_hash['username'],
|
|
:OS_PROJECT_NAME => credential_hash['project_name'],
|
|
:OS_PASSWORD => credential_hash['password'],
|
|
:OS_PROJECT_DOMAIN_NAME => credential_hash['project_domain_name'],
|
|
:OS_USER_DOMAIN_NAME => credential_hash['user_domain_name'],
|
|
}
|
|
klass.expects(:get_ironic_credentials).with().returns(credential_hash)
|
|
klass.expects(:withenv).with(authenv)
|
|
klass.auth_ironic('test_retries')
|
|
end
|
|
|
|
['[Errno 111] Connection refused',
|
|
'(HTTP 400)'].reverse.each do |valid_message|
|
|
it "should retry when ironic cli returns with error #{valid_message}" do
|
|
klass.expects(:get_ironic_credentials).with().returns({})
|
|
klass.expects(:sleep).with(10).returns(nil)
|
|
klass.expects(:ironic).twice.with(['test_retries']).raises(
|
|
Exception, valid_message).then.returns('')
|
|
klass.auth_ironic('test_retries')
|
|
end
|
|
end
|
|
|
|
end
|
|
|
|
describe 'when listing ironic resources' do
|
|
|
|
it 'should exclude the column header' do
|
|
output = <<-EOT
|
|
id
|
|
net1
|
|
net2
|
|
EOT
|
|
klass.expects(:auth_ironic).returns(output)
|
|
result = klass.list_ironic_resources('foo')
|
|
expect(result).to eql(['net1', 'net2'])
|
|
end
|
|
|
|
end
|
|
|
|
end
|