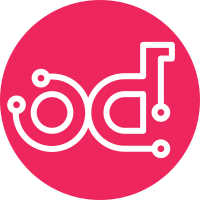
The client has been completely rewritten in order to use cliff. The code should be easier to maintain: authentication is now entirely handled by keystoneauth, CloudKitty's client and CK's OSC plugin use the exact same classes (no code duplication). New features for users: * Client-side CSV report generation: It is possible for users to generate CSV reports with the new client. There is a default format, but reports may also be configured through a yaml config file. (see documentation) * The documentation has been improved. (A few examples on how to use the python library + complete API bindings and CLI reference). * It is now possible to use the client without Keystone authentication (this requires that CK's API is configured to use the noauth auth strategy). * Various features are brought by cliff: completion, command output formatting (table, shell, yaml, json...). New features for developpers: * Python 2.7/3.5 compatible 'python-cloudkittyclient' module. * Integration tests (for 'openstack rating' and 'cloudkitty') have been added. These allow to create gate jobs running against a CK devstack * Tests are now ran with stestr instead of testr, which allows a better control over execution. * The dependency list has been reduced and upper constraints have been set. Change-Id: I7c6afa46138d499b37b8be3d049b23ab5302a928 Task: 6589 Story: 2001614
48 lines
1.5 KiB
Python
48 lines
1.5 KiB
Python
# -*- coding: utf-8 -*-
|
|
# Copyright 2018 Objectif Libre
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
#
|
|
from keystoneauth1 import loading
|
|
from keystoneauth1 import plugin
|
|
|
|
|
|
class CloudKittyNoAuthPlugin(plugin.BaseAuthPlugin):
|
|
"""No authentication plugin for CloudKitty
|
|
|
|
"""
|
|
def __init__(self, endpoint='http://localhost:8889', *args, **kwargs):
|
|
super(CloudKittyNoAuthPlugin, self).__init__()
|
|
self._endpoint = endpoint
|
|
|
|
def get_auth_ref(self, session, **kwargs):
|
|
return None
|
|
|
|
def get_endpoint(self, session, **kwargs):
|
|
return self._endpoint
|
|
|
|
def get_headers(self, session, **kwargs):
|
|
return {}
|
|
|
|
|
|
class CloudKittyNoAuthLoader(loading.BaseLoader):
|
|
plugin_class = CloudKittyNoAuthPlugin
|
|
|
|
def get_options(self):
|
|
options = super(CloudKittyNoAuthLoader, self).get_options()
|
|
options.extend([
|
|
loading.Opt('endpoint', help='CloudKitty Endpoint',
|
|
required=True, default='http://localhost:8889'),
|
|
])
|
|
return options
|