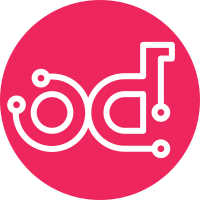
Replace usage of oslo-incubator modules strutils, importutils, timeutils with corresponding oslo.utils modules instead. Removes timeutils module, others will be replaced with next oslo-incubator sync. Change-Id: I12530f4e4d745f9a95b339182e31189e32e56fa6
86 lines
2.5 KiB
Python
86 lines
2.5 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from __future__ import print_function
|
|
|
|
import sys
|
|
|
|
from oslo.utils import encodeutils
|
|
import prettytable
|
|
import six
|
|
|
|
|
|
def _print(pt, order=None):
|
|
output = pt.get_string(sortby=order) if order else pt.get_string()
|
|
if sys.version_info >= (3, 0):
|
|
print(output)
|
|
else:
|
|
print(encodeutils.safe_encode(output))
|
|
|
|
|
|
def print_list(objs, fields, formatters={}, order_by=None):
|
|
mixed_case_fields = ['serverId']
|
|
pt = prettytable.PrettyTable([f for f in fields], caching=False)
|
|
pt.aligns = ['l' for f in fields]
|
|
|
|
for o in objs:
|
|
row = []
|
|
for field in fields:
|
|
if field in formatters:
|
|
row.append(formatters[field](o))
|
|
else:
|
|
if field in mixed_case_fields:
|
|
field_name = field.replace(' ', '_')
|
|
else:
|
|
field_name = field.lower().replace(' ', '_')
|
|
data = getattr(o, field_name, '')
|
|
row.append(data)
|
|
pt.add_row(row)
|
|
_print(pt, order_by)
|
|
|
|
|
|
def print_dict(d, property="Property"):
|
|
pt = prettytable.PrettyTable([property, 'Value'], caching=False)
|
|
pt.aligns = ['l', 'l']
|
|
[pt.add_row(list(r)) for r in six.iteritems(d)]
|
|
_print(pt, property)
|
|
|
|
|
|
class HookableMixin(object):
|
|
"""Mixin so classes can register and run hooks."""
|
|
_hooks_map = {}
|
|
|
|
@classmethod
|
|
def add_hook(cls, hook_type, hook_func):
|
|
if hook_type not in cls._hooks_map:
|
|
cls._hooks_map[hook_type] = []
|
|
|
|
cls._hooks_map[hook_type].append(hook_func)
|
|
|
|
@classmethod
|
|
def run_hooks(cls, hook_type, *args, **kwargs):
|
|
hook_funcs = cls._hooks_map.get(hook_type) or []
|
|
for hook_func in hook_funcs:
|
|
hook_func(*args, **kwargs)
|
|
|
|
|
|
def safe_issubclass(*args):
|
|
"""Like issubclass, but will just return False if not a class."""
|
|
|
|
try:
|
|
if issubclass(*args):
|
|
return True
|
|
except TypeError:
|
|
pass
|
|
|
|
return False
|