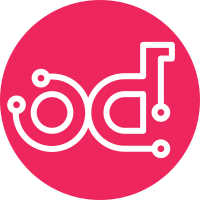
Change [1] introduced support of microversions to manilaclient and changed behaviour of client init that is not compatible with old approach. Also, not all old modules have deprecated modules in place. So, first problem description: - Old client expected string with api version, new one expects APIVersion class instance. So, add support for both and cover them with unit tests. Second problem description: - Manila UI project imports "manilaclient.v1.contrib.list_extensions" directly, but this file was moved to another place without keeping in place deprecated variant. So, add such to fix backward compatibility and allow to use newest manilaclient with manila-ui project. [1] I3733fe85424e39566addc070d42609e508259f19 Change-Id: I838631fb38b8ddf1f4f1e06269af122bf51a5155 Closes-Bug: #1518245
56 lines
1.9 KiB
Python
56 lines
1.9 KiB
Python
# Copyright 2010 Jacob Kaplan-Moss
|
|
# Copyright 2011 OpenStack LLC.
|
|
# Copyright 2011 Piston Cloud Computing, Inc.
|
|
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
OpenStack Client interface. Handles the REST calls and responses.
|
|
"""
|
|
|
|
from oslo_utils import importutils
|
|
|
|
from manilaclient import api_versions
|
|
from manilaclient import exceptions
|
|
|
|
|
|
def get_client_class(version):
|
|
version_map = {
|
|
'1': 'manilaclient.v1.client.Client',
|
|
'2': 'manilaclient.v2.client.Client',
|
|
}
|
|
try:
|
|
client_path = version_map[str(version)]
|
|
except (KeyError, ValueError):
|
|
msg = "Invalid client version '%s'. must be one of: %s" % (
|
|
(version, ', '.join(version_map)))
|
|
raise exceptions.UnsupportedVersion(msg)
|
|
|
|
return importutils.import_class(client_path)
|
|
|
|
|
|
def Client(api_version, *args, **kwargs):
|
|
if not hasattr(api_version, 'get_major_version'):
|
|
if api_version in ('1', '1.0'):
|
|
api_version = api_versions.APIVersion(
|
|
api_versions.DEPRECATED_VERSION)
|
|
elif api_version == '2':
|
|
api_version = api_versions.APIVersion(api_versions.MIN_VERSION)
|
|
else:
|
|
api_version = api_versions.APIVersion(api_version)
|
|
client_class = get_client_class(api_version.get_major_version())
|
|
kwargs['api_version'] = api_version
|
|
return client_class(*args, **kwargs)
|