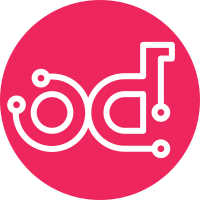
If no arguments are provided while executing neutron quota-update instead of printing the current quota values it will throw an error message "Must specify new values to update quota" Added unit test to verify that the exception is raised when no arguments are provided to quota update Includes release notes. DocImpact Closes-Bug: #1597552 Change-Id: I476991c39eafa16148f0cc3252ae5c26b9c8cbfc
103 lines
3.9 KiB
Python
103 lines
3.9 KiB
Python
#!/usr/bin/env python
|
|
# Copyright (C) 2013 Yahoo! Inc.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import sys
|
|
|
|
from mox3 import mox
|
|
|
|
from neutronclient.common import exceptions
|
|
from neutronclient.neutron.v2_0 import quota as test_quota
|
|
from neutronclient.tests.unit import test_cli20
|
|
|
|
|
|
class CLITestV20Quota(test_cli20.CLITestV20Base):
|
|
def test_show_quota(self):
|
|
resource = 'quota'
|
|
cmd = test_quota.ShowQuota(
|
|
test_cli20.MyApp(sys.stdout), None)
|
|
args = ['--tenant-id', self.test_id]
|
|
self._test_show_resource(resource, cmd, self.test_id, args)
|
|
|
|
def test_update_quota(self):
|
|
resource = 'quota'
|
|
cmd = test_quota.UpdateQuota(
|
|
test_cli20.MyApp(sys.stdout), None)
|
|
args = ['--tenant-id', self.test_id, '--network', 'test']
|
|
self.assertRaises(
|
|
exceptions.NeutronClientException, self._test_update_resource,
|
|
resource, cmd, self.test_id, args=args,
|
|
extrafields={'network': 'new'})
|
|
|
|
def test_delete_quota_get_parser(self):
|
|
cmd = test_cli20.MyApp(sys.stdout)
|
|
test_quota.DeleteQuota(cmd, None).get_parser(cmd)
|
|
|
|
def test_show_quota_positional(self):
|
|
resource = 'quota'
|
|
cmd = test_quota.ShowQuota(
|
|
test_cli20.MyApp(sys.stdout), None)
|
|
args = [self.test_id]
|
|
self._test_show_resource(resource, cmd, self.test_id, args)
|
|
|
|
def test_update_quota_positional(self):
|
|
resource = 'quota'
|
|
cmd = test_quota.UpdateQuota(
|
|
test_cli20.MyApp(sys.stdout), None)
|
|
args = [self.test_id, '--network', 'test']
|
|
self.assertRaises(
|
|
exceptions.NeutronClientException, self._test_update_resource,
|
|
resource, cmd, self.test_id, args=args,
|
|
extrafields={'network': 'new'})
|
|
|
|
def test_show_quota_default(self):
|
|
resource = 'quota'
|
|
cmd = test_quota.ShowQuotaDefault(
|
|
test_cli20.MyApp(sys.stdout), None)
|
|
args = ['--tenant-id', self.test_id]
|
|
self.mox.StubOutWithMock(cmd, "get_client")
|
|
self.mox.StubOutWithMock(self.client.httpclient, "request")
|
|
cmd.get_client().MultipleTimes().AndReturn(self.client)
|
|
expected_res = {'quota': {'port': 50, 'network': 10, 'subnet': 10}}
|
|
resstr = self.client.serialize(expected_res)
|
|
path = getattr(self.client, "quota_default_path")
|
|
return_tup = (test_cli20.MyResp(200), resstr)
|
|
self.client.httpclient.request(
|
|
test_cli20.end_url(path % self.test_id), 'GET',
|
|
body=None,
|
|
headers=mox.ContainsKeyValue(
|
|
'X-Auth-Token', test_cli20.TOKEN)).AndReturn(return_tup)
|
|
self.mox.ReplayAll()
|
|
|
|
cmd_parser = cmd.get_parser("test_" + resource)
|
|
parsed_args = cmd_parser.parse_args(args)
|
|
cmd.run(parsed_args)
|
|
|
|
self.mox.VerifyAll()
|
|
self.mox.UnsetStubs()
|
|
_str = self.fake_stdout.make_string()
|
|
self.assertIn('network', _str)
|
|
self.assertIn('subnet', _str)
|
|
self.assertIn('port', _str)
|
|
self.assertNotIn('subnetpool', _str)
|
|
|
|
def test_update_quota_noargs(self):
|
|
resource = 'quota'
|
|
cmd = test_quota.UpdateQuota(test_cli20.MyApp(sys.stdout), None)
|
|
args = [self.test_id]
|
|
self.assertRaises(exceptions.CommandError, self._test_update_resource,
|
|
resource, cmd, self.test_id, args=args,
|
|
extrafields=None)
|