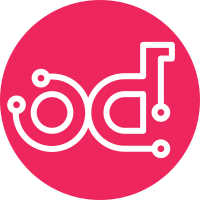
The first part of adding flavor support for the Nova V3 API (support for flavor-access API calls is still to come). Restructures v1_1 testcases so as much as possible so they can be reused for the V3 version of the tests. If it looks too ugly an alternative would be to just cut and paste the fakes and test from v1_1 to v3, which is simpler to understand but comes at the cost of duplicated code. The upside though is it would be much easier to remove v1_1/v2 support in the future. Partially implements blueprint v3-api Change-Id: Ic7cb3c43db02c07d37aea2675b310aaa50639c40
100 lines
3.0 KiB
Python
100 lines
3.0 KiB
Python
# Copyright 2010 Jacob Kaplan-Moss
|
|
# Copyright 2013 IBM Corp.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
Flavor interface.
|
|
"""
|
|
from novaclient import base
|
|
from novaclient.v1_1 import flavors
|
|
|
|
|
|
class Flavor(base.Resource):
|
|
"""
|
|
A flavor is an available hardware configuration for a server.
|
|
"""
|
|
HUMAN_ID = True
|
|
|
|
def __repr__(self):
|
|
return "<Flavor: %s>" % self.name
|
|
|
|
@property
|
|
def is_public(self):
|
|
"""
|
|
Provide a user-friendly accessor to flavor-access:is_public
|
|
"""
|
|
return self._info.get("flavor-access:is_public", 'N/A')
|
|
|
|
def get_keys(self):
|
|
"""
|
|
Get extra specs from a flavor.
|
|
|
|
:param flavor: The :class:`Flavor` to get extra specs from
|
|
"""
|
|
_resp, body = self.manager.api.client.get(
|
|
"/flavors/%s/flavor-extra-specs" %
|
|
base.getid(self))
|
|
return body["extra_specs"]
|
|
|
|
def set_keys(self, metadata):
|
|
"""
|
|
Set extra specs on a flavor.
|
|
|
|
:param flavor: The :class:`Flavor` to set extra spec on
|
|
:param metadata: A dict of key/value pairs to be set
|
|
"""
|
|
body = {'extra_specs': metadata}
|
|
return self.manager._create(
|
|
"/flavors/%s/flavor-extra-specs" %
|
|
base.getid(self), body, "extra_specs",
|
|
return_raw=True)
|
|
|
|
def unset_keys(self, keys):
|
|
"""
|
|
Unset extra specs on a flavor.
|
|
|
|
:param flavor: The :class:`Flavor` to unset extra spec on
|
|
:param keys: A list of keys to be unset
|
|
"""
|
|
for k in keys:
|
|
return self.manager._delete(
|
|
"/flavors/%s/flavor-extra-specs/%s" % (
|
|
base.getid(self), k))
|
|
|
|
def delete(self):
|
|
"""
|
|
Delete this flavor.
|
|
"""
|
|
self.manager.delete(self)
|
|
|
|
|
|
class FlavorManager(flavors.FlavorManager):
|
|
resource_class = Flavor
|
|
|
|
def _build_body(self, name, ram, vcpus, disk, id, swap,
|
|
ephemeral, rxtx_factor, is_public):
|
|
return {
|
|
"flavor": {
|
|
"name": name,
|
|
"ram": ram,
|
|
"vcpus": vcpus,
|
|
"disk": disk,
|
|
"id": id,
|
|
"swap": swap,
|
|
"ephemeral": ephemeral,
|
|
"rxtx_factor": rxtx_factor,
|
|
"flavor-access:is_public": is_public,
|
|
}
|
|
}
|