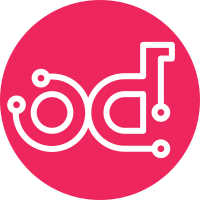
There are 2 known issues which breaks token auth method in CLI: * The wrong check of flag (the check should be for --os-token since arguments are not parsed at that moment) is performed in CLI inner method `_append_global_identity_args`. It led to usage of "password" auth type by default[1] even if `--os-token` cli argument is specified. If `--os-auth-type` is specified to token, keystoneauth1 library makes the right decision[2]. * Based on an auth type, keystoneauth library registers different CLI arguments[3]. It means that `--os-username` argument is available only in password auth type, `--os-token` is available only in token auth type, etc. It also affects the way in which the python code should access such arguments. The arguments which are unrelated to the selected auth type are omitted from the parsed arguments object. That sounds reasonable, but unfortunately the code assumes the unrelated arguments are always present which leads to an AttributeError. Combination of these 2 issues made token auth type broken in CLI layer. [1]ee2221f052/novaclient/shell.py (L255-L257)
[2]14dd37b34c/keystoneauth1/loading/cli.py (L51-L52)
[3]14dd37b34c/keystoneauth1/loading/cli.py (L65-L73)
Closes-Bug: #1659015 Change-Id: Ibc861d396b71fe105288d8336623cc22cf92523e
81 lines
3.3 KiB
Python
81 lines
3.3 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from six.moves.urllib import parse
|
|
import tempest.lib.cli.base
|
|
|
|
from novaclient import client
|
|
from novaclient.tests.functional import base
|
|
|
|
|
|
class TestAuthentication(base.ClientTestBase):
|
|
|
|
def _get_url(self, identity_api_version):
|
|
url = parse.urlparse(self.cli_clients.uri)
|
|
return parse.urlunparse((url.scheme, url.netloc,
|
|
'/identity/v%s' % identity_api_version,
|
|
url.params, url.query,
|
|
url.fragment))
|
|
|
|
def nova_auth_with_password(self, action, identity_api_version):
|
|
flags = ('--os-username %s --os-tenant-name %s --os-password %s '
|
|
'--os-auth-url %s --os-endpoint-type publicURL' % (
|
|
self.cli_clients.username,
|
|
self.cli_clients.tenant_name,
|
|
self.cli_clients.password,
|
|
self._get_url(identity_api_version)))
|
|
if self.cli_clients.insecure:
|
|
flags += ' --insecure '
|
|
|
|
return tempest.lib.cli.base.execute(
|
|
"nova", action, flags, cli_dir=self.cli_clients.cli_dir)
|
|
|
|
def nova_auth_with_token(self, identity_api_version):
|
|
auth_ref = self.client.client.session.auth.get_access(
|
|
self.client.client.session)
|
|
token = auth_ref.auth_token
|
|
auth_url = self._get_url(identity_api_version)
|
|
kw = {}
|
|
if identity_api_version == "3":
|
|
kw["project_domain_id"] = self.project_domain_id
|
|
nova = client.Client("2", auth_token=token, auth_url=auth_url,
|
|
project_name=self.project_name, **kw)
|
|
nova.servers.list()
|
|
|
|
flags = ('--os-tenant-name %(project_name)s --os-token %(token)s '
|
|
'--os-auth-url %(auth_url)s --os-endpoint-type publicURL'
|
|
% {"project_name": self.project_name,
|
|
"token": token, "auth_url": auth_url})
|
|
if self.cli_clients.insecure:
|
|
flags += ' --insecure '
|
|
|
|
tempest.lib.cli.base.execute(
|
|
"nova", "list", flags, cli_dir=self.cli_clients.cli_dir)
|
|
|
|
def test_auth_via_keystone_v2(self):
|
|
session = self.keystone.session
|
|
version = (2, 0)
|
|
if not base.is_keystone_version_available(session, version):
|
|
self.skipTest("Identity API version 2.0 is not available.")
|
|
|
|
self.nova_auth_with_password("list", identity_api_version="2.0")
|
|
self.nova_auth_with_token(identity_api_version="2.0")
|
|
|
|
def test_auth_via_keystone_v3(self):
|
|
session = self.keystone.session
|
|
version = (3, 0)
|
|
if not base.is_keystone_version_available(session, version):
|
|
self.skipTest("Identity API version 3.0 is not available.")
|
|
|
|
self.nova_auth_with_password("list", identity_api_version="3")
|
|
self.nova_auth_with_token(identity_api_version="3")
|