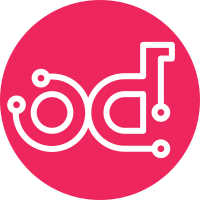
Add return-request-id-to-caller function to resources and resource managers in the following files. The methods in the resource class and resource manager return a wrapper class that has 'request_ids' property. The caller can get request ids of the callee via the property. * novaclient/v2/keypairs.py * novaclient/v2/limits.py * novaclient/v2/networks.py * novaclient/v2/quota_classes.py * novaclient/v2/quotas.py * novaclient/v2/security_group_default_rules.py * novaclient/v2/security_group_rules.py * novaclient/v2/security_groups.py * novaclient/v2/server_groups.py * novaclient/v2/services.py * novaclient/v2/usage.py * novaclient/v2/versions.py Co-authored-by: Ankit Agrawal <ankit11.agrawal@nttdata.com> Change-Id: I9203f70a0eef5686b590fbff35563f2cf8b6f586 Implements: blueprint return-request-id-to-caller
107 lines
3.2 KiB
Python
107 lines
3.2 KiB
Python
# Copyright 2011 OpenStack Foundation
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
Security group interface (1.1 extension).
|
|
"""
|
|
|
|
import six
|
|
from six.moves.urllib import parse
|
|
|
|
from novaclient import base
|
|
|
|
|
|
class SecurityGroup(base.Resource):
|
|
def __str__(self):
|
|
return str(self.id)
|
|
|
|
def delete(self):
|
|
"""
|
|
Delete this security group.
|
|
|
|
:returns: An instance of novaclient.base.TupleWithMeta
|
|
"""
|
|
return self.manager.delete(self)
|
|
|
|
def update(self):
|
|
"""
|
|
Update this security group.
|
|
|
|
:returns: :class:`SecurityGroup`
|
|
"""
|
|
return self.manager.update(self)
|
|
|
|
|
|
class SecurityGroupManager(base.ManagerWithFind):
|
|
resource_class = SecurityGroup
|
|
|
|
def create(self, name, description):
|
|
"""
|
|
Create a security group
|
|
|
|
:param name: name for the security group to create
|
|
:param description: description of the security group
|
|
:rtype: the security group object
|
|
"""
|
|
body = {"security_group": {"name": name, 'description': description}}
|
|
return self._create('/os-security-groups', body, 'security_group')
|
|
|
|
def update(self, group, name, description):
|
|
"""
|
|
Update a security group
|
|
|
|
:param group: The security group to update (group or ID)
|
|
:param name: name for the security group to update
|
|
:param description: description for the security group to update
|
|
:rtype: the security group object
|
|
"""
|
|
body = {"security_group": {"name": name, 'description': description}}
|
|
return self._update('/os-security-groups/%s' % base.getid(group),
|
|
body, 'security_group')
|
|
|
|
def delete(self, group):
|
|
"""
|
|
Delete a security group
|
|
|
|
:param group: The security group to delete (group or ID)
|
|
:returns: An instance of novaclient.base.TupleWithMeta
|
|
"""
|
|
return self._delete('/os-security-groups/%s' % base.getid(group))
|
|
|
|
def get(self, group_id):
|
|
"""
|
|
Get a security group
|
|
|
|
:param group_id: The security group to get by ID
|
|
:rtype: :class:`SecurityGroup`
|
|
"""
|
|
return self._get('/os-security-groups/%s' % group_id,
|
|
'security_group')
|
|
|
|
def list(self, search_opts=None):
|
|
"""
|
|
Get a list of all security_groups
|
|
|
|
:rtype: list of :class:`SecurityGroup`
|
|
"""
|
|
search_opts = search_opts or {}
|
|
|
|
qparams = dict((k, v) for (k, v) in six.iteritems(search_opts) if v)
|
|
|
|
query_string = '?%s' % parse.urlencode(qparams) if qparams else ''
|
|
|
|
return self._list('/os-security-groups%s' % query_string,
|
|
'security_groups')
|