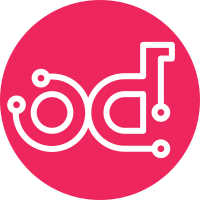
These were deprecated in Newton:
aaebeb05a0
Since this is the last of the deprecated contrib extensions,
we can also deprecate the 'only_contrib' parameter from the
novaclient.client.discover_extensions method.
Change-Id: Ie2e3fdc4e044f6eb304724d16a7d0f1f7ba705fd
73 lines
2.5 KiB
Python
73 lines
2.5 KiB
Python
# Copyright 2012 OpenStack Foundation
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import imp
|
|
import inspect
|
|
|
|
import mock
|
|
import pkg_resources
|
|
|
|
from novaclient import client
|
|
from novaclient.tests.unit import utils
|
|
|
|
|
|
class DiscoverTest(utils.TestCase):
|
|
|
|
def test_discover_via_entry_points(self):
|
|
|
|
def mock_iter_entry_points(group):
|
|
if group == 'novaclient.extension':
|
|
fake_ep = mock.Mock()
|
|
fake_ep.name = 'foo'
|
|
fake_ep.module = imp.new_module('foo')
|
|
fake_ep.load.return_value = fake_ep.module
|
|
return [fake_ep]
|
|
|
|
@mock.patch.object(pkg_resources, 'iter_entry_points',
|
|
mock_iter_entry_points)
|
|
def test():
|
|
for name, module in client._discover_via_entry_points():
|
|
self.assertEqual('foo', name)
|
|
self.assertTrue(inspect.ismodule(module))
|
|
|
|
test()
|
|
|
|
def test_discover_extensions(self):
|
|
|
|
def mock_discover_via_python_path():
|
|
yield 'foo', imp.new_module('foo')
|
|
|
|
def mock_discover_via_entry_points():
|
|
yield 'baz', imp.new_module('baz')
|
|
|
|
@mock.patch.object(client,
|
|
'_discover_via_python_path',
|
|
mock_discover_via_python_path)
|
|
@mock.patch.object(client,
|
|
'_discover_via_entry_points',
|
|
mock_discover_via_entry_points)
|
|
def test():
|
|
extensions = client.discover_extensions('1.1')
|
|
self.assertEqual(2, len(extensions))
|
|
names = sorted(['foo', 'baz'])
|
|
sorted_extensions = sorted(extensions, key=lambda ext: ext.name)
|
|
for i in range(len(names)):
|
|
ext = sorted_extensions[i]
|
|
name = names[i]
|
|
self.assertEqual(ext.name, name)
|
|
self.assertTrue(inspect.ismodule(ext.module))
|
|
|
|
test()
|