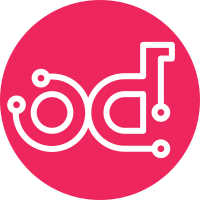
This change is entirely automated save for the update of some mocks from 'io.open' to '__builtins__.open'). We are keeping this change separate from addition of the actual hook so that we can ignore the commit later. Change-Id: I0a9d8736632084473b57b57b693322447d7be519 Signed-off-by: Stephen Finucane <stephenfin@redhat.com>
72 lines
2.3 KiB
Python
72 lines
2.3 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
#
|
|
|
|
"""Configuration action implementations"""
|
|
|
|
from keystoneauth1.loading import base
|
|
from osc_lib.command import command
|
|
|
|
from openstackclient.i18n import _
|
|
|
|
REDACTED = "<redacted>"
|
|
|
|
|
|
class ShowConfiguration(command.ShowOne):
|
|
_description = _("Display configuration details")
|
|
|
|
auth_required = False
|
|
|
|
def get_parser(self, prog_name):
|
|
parser = super().get_parser(prog_name)
|
|
mask_group = parser.add_mutually_exclusive_group()
|
|
mask_group.add_argument(
|
|
"--mask",
|
|
dest="mask",
|
|
action="store_true",
|
|
default=True,
|
|
help=_("Attempt to mask passwords (default)"),
|
|
)
|
|
mask_group.add_argument(
|
|
"--unmask",
|
|
dest="mask",
|
|
action="store_false",
|
|
help=_("Show password in clear text"),
|
|
)
|
|
return parser
|
|
|
|
def take_action(self, parsed_args):
|
|
info = self.app.client_manager.get_configuration()
|
|
|
|
# Assume a default secret list in case we do not have an auth_plugin
|
|
secret_opts = ["password", "token"]
|
|
|
|
if getattr(self.app.client_manager, "auth_plugin_name", None):
|
|
auth_plg_name = self.app.client_manager.auth_plugin_name
|
|
secret_opts = [
|
|
o.dest
|
|
for o in base.get_plugin_options(auth_plg_name)
|
|
if o.secret
|
|
]
|
|
|
|
for key, value in info.pop('auth', {}).items():
|
|
if parsed_args.mask and key.lower() in secret_opts:
|
|
value = REDACTED
|
|
info['auth.' + key] = value
|
|
|
|
if parsed_args.mask:
|
|
for secret_opt in secret_opts:
|
|
if secret_opt in info:
|
|
info[secret_opt] = REDACTED
|
|
|
|
return zip(*sorted(info.items()))
|