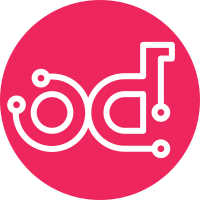
Patch adds verification of resuls of creation and list actions. Patch contains changes in both scenarios and unit tests and affect on following scenarios: rally/plugins/openstack/scenarios/ceilometer/events.py rally/plugins/openstack/scenarios/ceilometer/resources.py rally/plugins/openstack/scenarios/designate/basic.py rally/plugins/openstack/scenarios/glance/images.py rally/plugins/openstack/scenarios/heat/stacks.py rally/plugins/openstack/scenarios/ironic/nodes.py rally/plugins/openstack/scenarios/keystone/basic.py rally/plugins/openstack/scenarios/magnum/clusters.py rally/plugins/openstack/scenarios/manila/shares.py Change-Id: I5b0156c809cc4be3e265198e64fea1e9251c78a9
111 lines
4.3 KiB
Python
111 lines
4.3 KiB
Python
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import ddt
|
|
import mock
|
|
|
|
from rally import exceptions
|
|
from rally.plugins.openstack.scenarios.magnum import clusters
|
|
from tests.unit import test
|
|
|
|
|
|
@ddt.ddt
|
|
class MagnumClustersTestCase(test.ScenarioTestCase):
|
|
|
|
@staticmethod
|
|
def _get_context():
|
|
context = test.get_test_context()
|
|
context.update({
|
|
"tenant": {
|
|
"id": "rally_tenant_id",
|
|
"cluster_template": "rally_cluster_template_uuid"
|
|
}
|
|
})
|
|
return context
|
|
|
|
@ddt.data(
|
|
{"kwargs": {}},
|
|
{"kwargs": {"fakearg": "f"}})
|
|
def test_list_clusters(self, kwargs):
|
|
scenario = clusters.ListClusters()
|
|
scenario._list_clusters = mock.Mock()
|
|
|
|
scenario.run(**kwargs)
|
|
|
|
scenario._list_clusters.assert_called_once_with(**kwargs)
|
|
|
|
def test_create_cluster_with_existing_ct_and_list_clusters(self):
|
|
scenario = clusters.CreateAndListClusters()
|
|
kwargs = {"cluster_template_uuid": "existing_cluster_template_uuid",
|
|
"fakearg": "f"}
|
|
fake_cluster = mock.Mock()
|
|
scenario._create_cluster = mock.Mock(return_value=fake_cluster)
|
|
scenario._list_clusters = mock.Mock(return_value=[fake_cluster,
|
|
mock.Mock(),
|
|
mock.Mock()])
|
|
|
|
# Positive case
|
|
scenario.run(2, **kwargs)
|
|
|
|
scenario._create_cluster.assert_called_once_with(
|
|
"existing_cluster_template_uuid", 2, **kwargs)
|
|
scenario._list_clusters.assert_called_once_with(**kwargs)
|
|
|
|
# Negative case1: cluster isn't created
|
|
scenario._create_cluster.return_value = None
|
|
self.assertRaises(exceptions.RallyAssertionError,
|
|
scenario.run, 2, **kwargs)
|
|
scenario._create_cluster.assert_called_with(
|
|
"existing_cluster_template_uuid", 2, **kwargs)
|
|
|
|
# Negative case2: created cluster not in the list of available clusters
|
|
scenario._create_cluster.return_value = mock.MagicMock()
|
|
self.assertRaises(exceptions.RallyAssertionError,
|
|
scenario.run, 2, **kwargs)
|
|
scenario._create_cluster.assert_called_with(
|
|
"existing_cluster_template_uuid", 2, **kwargs)
|
|
scenario._list_clusters.assert_called_with(**kwargs)
|
|
|
|
def test_create_and_list_clusters(self):
|
|
context = self._get_context()
|
|
scenario = clusters.CreateAndListClusters(context)
|
|
fake_cluster = mock.Mock()
|
|
kwargs = {"fakearg": "f"}
|
|
scenario._create_cluster = mock.Mock(return_value=fake_cluster)
|
|
scenario._list_clusters = mock.Mock(return_value=[fake_cluster,
|
|
mock.Mock(),
|
|
mock.Mock()])
|
|
|
|
# Positive case
|
|
scenario.run(2, **kwargs)
|
|
|
|
scenario._create_cluster.assert_called_once_with(
|
|
"rally_cluster_template_uuid", 2, **kwargs)
|
|
scenario._list_clusters.assert_called_once_with(**kwargs)
|
|
|
|
# Negative case1: cluster isn't created
|
|
scenario._create_cluster.return_value = None
|
|
self.assertRaises(exceptions.RallyAssertionError,
|
|
scenario.run, 2, **kwargs)
|
|
scenario._create_cluster.assert_called_with(
|
|
"rally_cluster_template_uuid", 2, **kwargs)
|
|
|
|
# Negative case2: created cluster not in the list of available clusters
|
|
scenario._create_cluster.return_value = mock.MagicMock()
|
|
self.assertRaises(exceptions.RallyAssertionError,
|
|
scenario.run, 2, **kwargs)
|
|
scenario._create_cluster.assert_called_with(
|
|
"rally_cluster_template_uuid", 2, **kwargs)
|
|
scenario._list_clusters.assert_called_with(**kwargs)
|