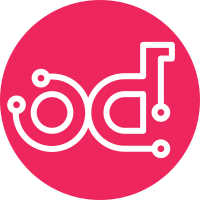
Some code cleanup for the recently merged https://review.openstack.org/111977 We took the atomic actions list by mistake always from the first iteration; we should continue taking this list from the first non-failed iteration. Add functional testing for this case. We also fix the missing "%" sign in the output CLI table in case there were no successful atomic action iterations. Co-authored-by: Oleg Anufriev <oanufriev@mirantis.com> Change-Id: Id4095122be8dd8bffeeaeabe407ff76c0f294686 Closes-Bug: 1372739 Closes-Bug: 1372998 Closes-Bug: 1373488 Closes-Bug: 1373576
71 lines
2.5 KiB
Python
71 lines
2.5 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
|
|
import mock
|
|
|
|
from rally.benchmark.scenarios.dummy import dummy
|
|
from rally import exceptions
|
|
from tests import test
|
|
|
|
|
|
class DummyTestCase(test.TestCase):
|
|
|
|
@mock.patch("rally.benchmark.scenarios.dummy.dummy.time")
|
|
def test_dummy(self, mock_sleep):
|
|
scenario = dummy.Dummy()
|
|
scenario.sleep_between = mock.MagicMock()
|
|
scenario.dummy()
|
|
self.assertFalse(mock_sleep.sleep.called)
|
|
|
|
scenario.dummy(sleep=10)
|
|
mock_sleep.sleep.assert_called_once_with(10)
|
|
|
|
def test_dummy_exception(self):
|
|
scenario = dummy.Dummy()
|
|
|
|
size_of_message = 5
|
|
self.assertRaises(exceptions.DummyScenarioException,
|
|
scenario.dummy_exception, size_of_message)
|
|
|
|
def test_dummy_exception_probability(self):
|
|
scenario = dummy.Dummy()
|
|
|
|
# should not raise an exception as probability is 0
|
|
for i in range(100):
|
|
scenario.dummy_exception_probability(exception_probability=0)
|
|
|
|
# should always raise an exception as probability is 1
|
|
for i in range(100):
|
|
self.assertRaises(exceptions.DummyScenarioException,
|
|
scenario.dummy_exception_probability,
|
|
{'exception_probability': 1})
|
|
|
|
def test_dummy_dummy_with_scenario_output(self):
|
|
scenario = dummy.Dummy()
|
|
result = scenario.dummy_with_scenario_output()
|
|
self.assertEqual(result['errors'], "")
|
|
# Since the data is generated in random,
|
|
# checking for not None
|
|
self.assertNotEqual(result['data'], None)
|
|
|
|
def test_dummy_random_fail_in_atomic(self):
|
|
scenario = dummy.Dummy()
|
|
|
|
for i in range(10):
|
|
scenario.dummy_random_fail_in_atomic(exception_probability=0)
|
|
|
|
for i in range(10):
|
|
self.assertRaises(KeyError,
|
|
scenario.dummy_random_fail_in_atomic,
|
|
{'exception_probability': 1})
|