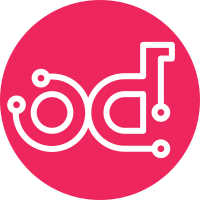
Back in a5e6f88e1
we made the assumption that repos containing puppet
and node.js modules that also have a setup.py were packaged as python
projects. That is not a valid assumption, so remove it. Projects can now
explicitly select between the packaging type.
Change-Id: Ie98b7677d07acdfaebf3fa725a481491101922eb
Signed-off-by: Doug Hellmann <doug@doughellmann.com>
39 lines
1.3 KiB
Python
39 lines
1.3 KiB
Python
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import json
|
|
import os.path
|
|
|
|
|
|
def looks_like_a_module(workdir, repo):
|
|
"Does the directory look like it contains a puppet module?"
|
|
if not os.path.exists(os.path.join(workdir, repo, 'metadata.json')):
|
|
return False
|
|
return any([
|
|
os.path.exists(os.path.join(workdir, repo, 'lib')),
|
|
os.path.exists(os.path.join(workdir, repo, 'manifests')),
|
|
])
|
|
|
|
|
|
def get_metadata(workdir, repo):
|
|
"Load the metadata.json file from the repo"
|
|
with open(os.path.join(workdir, repo, 'metadata.json'), 'r') as f:
|
|
body = f.read()
|
|
return json.loads(body)
|
|
|
|
|
|
def get_version(workdir, repo):
|
|
"Get the version string from the project metadata."
|
|
return get_metadata(workdir, repo).get('version')
|