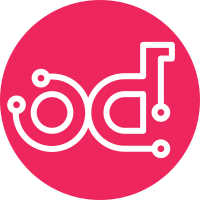
Change the 'std' release type to 'python-server' and add a 'python-pypi' release type for deliverables that are published to PyPI. Separate the release job validation from the validation of release version numbers and other settings to make the logic clearer. Add a new function to determine the release type for a project, either by checking the explicit value or guessing. Update the unit tests that relied on 'std'. Remove a unit test that tested a code path that has been removed. Change-Id: I704ec75fec61ecb6ee379239a5fa8612cb01b426 Signed-off-by: Doug Hellmann <doug@doughellmann.com>
52 lines
1.9 KiB
Python
52 lines
1.9 KiB
Python
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from oslotest import base
|
|
|
|
from openstack_releases import versionutils
|
|
|
|
|
|
class TestValidateVersion(base.BaseTestCase):
|
|
|
|
def test_valid_python_server(self):
|
|
errors = list(versionutils.validate_version('1.2.3'))
|
|
self.assertEqual(0, len(errors))
|
|
|
|
def test_invalid_python_server(self):
|
|
errors = list(versionutils.validate_version('1.2.3.4'))
|
|
self.assertEqual(1, len(errors))
|
|
|
|
def test_empty_python_server(self):
|
|
errors = list(versionutils.validate_version(''))
|
|
self.assertEqual(1, len(errors))
|
|
|
|
def test_valid_xstatic(self):
|
|
errors = list(versionutils.validate_version('1.2.3.4',
|
|
release_type='xstatic'))
|
|
self.assertEqual(0, len(errors))
|
|
|
|
def test_invalid_release_type(self):
|
|
errors = list(versionutils.validate_version('1.2.3',
|
|
release_type='nonesuch'))
|
|
self.assertEqual(1, len(errors))
|
|
|
|
def test_pre_not_allowed(self):
|
|
errors = list(versionutils.validate_version('1.2.3.0rc1',
|
|
pre_ok=False))
|
|
self.assertEqual(1, len(errors))
|
|
|
|
def test_pre_ok(self):
|
|
errors = list(versionutils.validate_version('1.2.3.0rc1'))
|
|
self.assertEqual(0, len(errors))
|