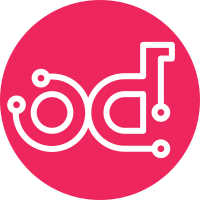
We had handling for renamed notes but not for deleted files. Add handling with tests. Change-Id: I20e40c6e60eee7840018abec07d23bc0be7cf696 Signed-off-by: Doug Hellmann <doug@doughellmann.com>
62 lines
2.0 KiB
Python
62 lines
2.0 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import binascii
|
|
import logging
|
|
import os
|
|
import os.path
|
|
import random
|
|
import subprocess
|
|
|
|
from reno import defaults
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
|
|
def get_notes_dir(args):
|
|
"""Return the path to the release notes directory."""
|
|
return os.path.join(args.relnotesdir, defaults.NOTES_SUBDIR)
|
|
|
|
|
|
def get_random_string(nbytes=8):
|
|
"""Return a fixed-length random string
|
|
|
|
:rtype: six.text_type
|
|
"""
|
|
try:
|
|
# NOTE(dhellmann): Not all systems support urandom().
|
|
# hexlify returns six.binary_type, decode to convert to six.text_type.
|
|
val = binascii.hexlify(os.urandom(nbytes)).decode('utf-8')
|
|
except Exception as e:
|
|
print('ERROR, perhaps urandom is not supported: %s' % e)
|
|
val = u''.join(u'%02x' % random.randrange(256)
|
|
for i in range(nbytes))
|
|
return val
|
|
|
|
|
|
def check_output(*args, **kwds):
|
|
"""Unicode-aware wrapper for subprocess.check_output"""
|
|
process = subprocess.Popen(stdout=subprocess.PIPE,
|
|
stderr=subprocess.PIPE,
|
|
*args, **kwds)
|
|
output, errors = process.communicate()
|
|
retcode = process.poll()
|
|
if errors:
|
|
LOG.debug('ran: %s', ' '.join(*args))
|
|
LOG.debug('returned: %s', retcode)
|
|
LOG.debug('error output: %s', errors.rstrip())
|
|
LOG.debug('regular output: %s', output.rstrip())
|
|
if retcode:
|
|
LOG.debug('raising error')
|
|
raise subprocess.CalledProcessError(retcode, args, output=output)
|
|
return output.decode('utf-8')
|