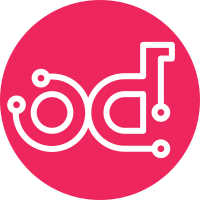
Some constraints files[1] contain valid data but we want to ignore them when generating a combined lower-constraints.txt, rather than erroring out: [tony@thor requirements]$ time .tox/venv/bin/build-lower-constraints ../*/lower-constraints.txt > tjmaxx.txt Traceback (most recent call last): File ".tox/venv/bin/build-lower-constraints", line 10, in <module> sys.exit(main()) File "/home/tony/projects/openstack/openstack/requirements/openstack_requirements/cmds/build_lower_constraints.py", line 71, in main merged = list(merge_constraints_sets(constraints_sets)) File "/home/tony/projects/openstack/openstack/requirements/openstack_requirements/cmds/build_lower_constraints.py", line 53, in merge_constraints_sets val = max((c[0] for c in constraints), key=get_requirements_version) File "/home/tony/projects/openstack/openstack/requirements/openstack_requirements/cmds/build_lower_constraints.py", line 43, in get_requirements_version raise ValueError('could not find version for {}'.format(req)) ValueError: could not find version for Requirement(package='', location='', specifiers='', markers='', comment='# flake8==2.5.5', extras=frozenset()) Let's just ignore comments in those files. [1] http://git.openstack.org/cgit/openstack/zaqar/tree/lower-constraints.txt#n28 Change-Id: Ie347ab273a1b239d9d264704482d3202dc4e4c74
70 lines
2.1 KiB
Python
70 lines
2.1 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import testtools
|
|
|
|
from openstack_requirements.cmds import build_lower_constraints
|
|
from openstack_requirements import requirement
|
|
|
|
|
|
class BuildLowerConstraintsTest(testtools.TestCase):
|
|
|
|
def test_one_input_file(self):
|
|
inputs = [
|
|
requirement.parse('package==1.2.3'),
|
|
]
|
|
expected = [
|
|
'package==1.2.3\n',
|
|
]
|
|
self.assertEqual(
|
|
expected,
|
|
list(build_lower_constraints.merge_constraints_sets(inputs))
|
|
)
|
|
|
|
def test_two_input_file_same(self):
|
|
inputs = [
|
|
requirement.parse('package==1.2.3'),
|
|
requirement.parse('package==1.2.3'),
|
|
]
|
|
expected = [
|
|
'package==1.2.3\n',
|
|
]
|
|
self.assertEqual(
|
|
expected,
|
|
list(build_lower_constraints.merge_constraints_sets(inputs))
|
|
)
|
|
|
|
def test_two_input_file_differ(self):
|
|
inputs = [
|
|
requirement.parse('package==1.2.3'),
|
|
requirement.parse('package==4.5.6'),
|
|
]
|
|
expected = [
|
|
'package==4.5.6\n',
|
|
]
|
|
self.assertEqual(
|
|
expected,
|
|
list(build_lower_constraints.merge_constraints_sets(inputs))
|
|
)
|
|
|
|
def test_one_input_file_with_comments(self):
|
|
inputs = [
|
|
requirement.parse('package==1.2.3\n # package2==0.9.8'),
|
|
]
|
|
expected = [
|
|
'package==1.2.3\n',
|
|
]
|
|
self.assertEqual(
|
|
expected,
|
|
list(build_lower_constraints.merge_constraints_sets(inputs))
|
|
)
|