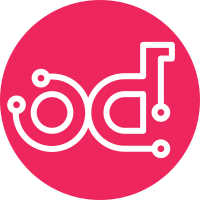
It's possible enabling OpenStack things can cause other images other than cirros to be made available (looking at you Magnum). We should make sure our Ansible tests only look for Cirros. Change-Id: Iefc01f8033629937552861366b9ddaac8058b249
95 lines
2.6 KiB
Bash
Executable File
95 lines
2.6 KiB
Bash
Executable File
#!/bin/bash
|
|
#############################################################################
|
|
# run-ansible-tests.sh
|
|
#
|
|
# Script used to setup a tox environment for running Ansible. This is meant
|
|
# to be called by tox (via tox.ini). To run the Ansible tests, use:
|
|
#
|
|
# tox -e ansible [TAG ...]
|
|
# or
|
|
# tox -e ansible -- -c cloudX [TAG ...]
|
|
# or to use the development version of Ansible:
|
|
# tox -e ansible -- -d -c cloudX [TAG ...]
|
|
#
|
|
# USAGE:
|
|
# run-ansible-tests.sh -e ENVDIR [-d] [-c CLOUD] [TAG ...]
|
|
#
|
|
# PARAMETERS:
|
|
# -d Use Ansible source repo development branch.
|
|
# -e ENVDIR Directory of the tox environment to use for testing.
|
|
# -c CLOUD Name of the cloud to use for testing.
|
|
# Defaults to "devstack-admin".
|
|
# [TAG ...] Optional list of space-separated tags to control which
|
|
# modules are tested.
|
|
#
|
|
# EXAMPLES:
|
|
# # Run all Ansible tests
|
|
# run-ansible-tests.sh -e ansible
|
|
#
|
|
# # Run auth, keypair, and network tests against cloudX
|
|
# run-ansible-tests.sh -e ansible -c cloudX auth keypair network
|
|
#############################################################################
|
|
|
|
|
|
CLOUD="devstack-admin"
|
|
ENVDIR=
|
|
USE_DEV=0
|
|
|
|
while getopts "c:de:" opt
|
|
do
|
|
case $opt in
|
|
d) USE_DEV=1 ;;
|
|
c) CLOUD=${OPTARG} ;;
|
|
e) ENVDIR=${OPTARG} ;;
|
|
?) echo "Invalid option: -${OPTARG}"
|
|
exit 1;;
|
|
esac
|
|
done
|
|
|
|
if [ -z ${ENVDIR} ]
|
|
then
|
|
echo "Option -e is required"
|
|
exit 1
|
|
fi
|
|
|
|
shift $((OPTIND-1))
|
|
TAGS=$( echo "$*" | tr ' ' , )
|
|
|
|
# We need to source the current tox environment so that Ansible will
|
|
# be setup for the correct python environment.
|
|
source $ENVDIR/bin/activate
|
|
|
|
if [ ${USE_DEV} -eq 1 ]
|
|
then
|
|
if [ -d ${ENVDIR}/ansible ]
|
|
then
|
|
echo "Using existing Ansible source repo"
|
|
else
|
|
echo "Installing Ansible source repo at $ENVDIR"
|
|
git clone --recursive git://github.com/ansible/ansible.git ${ENVDIR}/ansible
|
|
fi
|
|
source $ENVDIR/ansible/hacking/env-setup
|
|
else
|
|
echo "Installing Ansible from pip"
|
|
pip install ansible
|
|
fi
|
|
|
|
# Run the shade Ansible tests
|
|
tag_opt=""
|
|
if [ ! -z ${TAGS} ]
|
|
then
|
|
tag_opt="--tags ${TAGS}"
|
|
fi
|
|
|
|
# Until we have a module that lets us determine the image we want from
|
|
# within a playbook, we have to find the image here and pass it in.
|
|
# We use the openstack client instead of nova client since it can use clouds.yaml.
|
|
IMAGE=`openstack --os-cloud=${CLOUD} image list -f value -c Name | grep cirros | grep -v -e ramdisk -e kernel`
|
|
if [ $? -ne 0 ]
|
|
then
|
|
echo "Failed to find Cirros image"
|
|
exit 1
|
|
fi
|
|
|
|
ansible-playbook -vvv ./shade/tests/ansible/run.yml -e "cloud=${CLOUD} image=${IMAGE}" ${tag_opt}
|