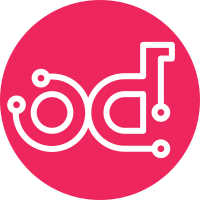
1. Add Chinese development documents 2. Add Chinese test document Change-Id: I29cd91f47069c9e64928800094282d4cbc977920
101 KiB
Executable File
1920x903px
101 KiB
Executable File
1920x903px
