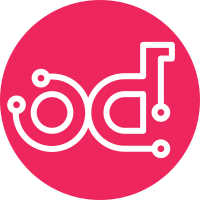
Currently, our integrity checking for objects is pretty weak when it comes to object metadata. If the extended attributes on a .data or .meta file get corrupted in such a way that we can still unpickle it, we don't have anything that detects that. This could be especially bad with encrypted etags; if the encrypted etag (X-Object-Sysmeta-Crypto-Etag or whatever it is) gets some bits flipped, then we'll cheerfully decrypt the cipherjunk into plainjunk, then send it to the client. Net effect is that the client sees a GET response with an ETag that doesn't match the MD5 of the object *and* Swift has no way of detecting and quarantining this object. Note that, with an unencrypted object, if the ETag metadatum gets mangled, then the object will be quarantined by the object server or auditor, whichever notices first. As part of this commit, I also ripped out some mocking of getxattr/setxattr in tests. It appears to be there to allow unit tests to run on systems where /tmp doesn't support xattrs. However, since the mock is keyed off of inode number and inode numbers get re-used, there's lots of leakage between different test runs. On a real FS, unlinking a file and then creating a new one of the same name will also reset the xattrs; this isn't the case with the mock. The mock was pretty old; Ubuntu 12.04 and up all support xattrs in /tmp, and recent Red Hat / CentOS releases do too. The xattr mock was added in 2011; maybe it was to support Ubuntu Lucid Lynx? Bonus: now you can pause a test with the debugger, inspect its files in /tmp, and actually see the xattrs along with the data. Since this patch now uses a real filesystem for testing filesystem operations, tests are skipped if the underlying filesystem does not support setting xattrs (eg tmpfs or more than 4k of xattrs on ext4). References to "/tmp" have been replaced with calls to tempfile.gettempdir(). This will allow setting the TMPDIR envvar in test setup and getting an XFS filesystem instead of ext4 or tmpfs. THIS PATCH SIGNIFICANTLY CHANGES TESTING ENVIRONMENTS With this patch, every test environment will require TMPDIR to be using a filesystem that supports at least 4k of extended attributes. Neither ext4 nor tempfs support this. XFS is recommended. So why all the SkipTests? Why not simply raise an error? We still need the tests to run on the base image for OpenStack's CI system. Since we were previously mocking out xattr, there wasn't a problem, but we also weren't actually testing anything. This patch adds functionality to validate xattr data, so we need to drop the mock. `test.unit.skip_if_no_xattrs()` is also imported into `test.functional` so that functional tests can import it from the functional test namespace. The related OpenStack CI infrastructure changes are made in https://review.openstack.org/#/c/394600/. Co-Authored-By: John Dickinson <me@not.mn> Change-Id: I98a37c0d451f4960b7a12f648e4405c6c6716808
105 lines
3.4 KiB
Python
105 lines
3.4 KiB
Python
# Copyright (c) 2016 OpenStack Foundation
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
|
|
# implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
'''Tests for `swift.common.linkat`'''
|
|
|
|
import ctypes
|
|
import unittest
|
|
import os
|
|
import mock
|
|
from uuid import uuid4
|
|
from tempfile import gettempdir
|
|
|
|
from swift.common.linkat import linkat
|
|
from swift.common.utils import O_TMPFILE
|
|
|
|
from test.unit import requires_o_tmpfile_support
|
|
|
|
|
|
class TestLinkat(unittest.TestCase):
|
|
|
|
def test_flags(self):
|
|
self.assertTrue(hasattr(linkat, 'AT_FDCWD'))
|
|
self.assertTrue(hasattr(linkat, 'AT_SYMLINK_FOLLOW'))
|
|
|
|
@mock.patch('swift.common.linkat.linkat._c_linkat', None)
|
|
def test_available(self):
|
|
self.assertFalse(linkat.available)
|
|
|
|
@requires_o_tmpfile_support
|
|
def test_errno(self):
|
|
with open('/dev/null', 'r') as fd:
|
|
self.assertRaises(IOError, linkat,
|
|
linkat.AT_FDCWD, "/proc/self/fd/%s" % (fd),
|
|
linkat.AT_FDCWD, "%s/testlinkat" % gettempdir(),
|
|
linkat.AT_SYMLINK_FOLLOW)
|
|
self.assertEqual(ctypes.get_errno(), 0)
|
|
|
|
@mock.patch('swift.common.linkat.linkat._c_linkat', None)
|
|
def test_unavailable(self):
|
|
self.assertRaises(EnvironmentError, linkat, 0, None, 0, None, 0)
|
|
|
|
def test_unavailable_in_libc(self):
|
|
|
|
class LibC(object):
|
|
|
|
def __init__(self):
|
|
self.linkat_retrieved = False
|
|
|
|
@property
|
|
def linkat(self):
|
|
self.linkat_retrieved = True
|
|
raise AttributeError
|
|
|
|
libc = LibC()
|
|
mock_cdll = mock.Mock(return_value=libc)
|
|
|
|
with mock.patch('ctypes.CDLL', new=mock_cdll):
|
|
# Force re-construction of a `Linkat` instance
|
|
# Something you're not supposed to do in actual code
|
|
new_linkat = type(linkat)()
|
|
self.assertFalse(new_linkat.available)
|
|
|
|
libc_name = ctypes.util.find_library('c')
|
|
|
|
mock_cdll.assert_called_once_with(libc_name, use_errno=True)
|
|
self.assertTrue(libc.linkat_retrieved)
|
|
|
|
@requires_o_tmpfile_support
|
|
def test_linkat_success(self):
|
|
|
|
fd = None
|
|
path = None
|
|
ret = -1
|
|
try:
|
|
fd = os.open(gettempdir(), O_TMPFILE | os.O_WRONLY)
|
|
path = os.path.join(gettempdir(), uuid4().hex)
|
|
ret = linkat(linkat.AT_FDCWD, "/proc/self/fd/%d" % (fd),
|
|
linkat.AT_FDCWD, path, linkat.AT_SYMLINK_FOLLOW)
|
|
self.assertEqual(ret, 0)
|
|
self.assertTrue(os.path.exists(path))
|
|
finally:
|
|
if fd:
|
|
os.close(fd)
|
|
if path and ret == 0:
|
|
# if linkat succeeded, remove file
|
|
os.unlink(path)
|
|
|
|
@mock.patch('swift.common.linkat.linkat._c_linkat')
|
|
def test_linkat_fd_not_integer(self, _mock_linkat):
|
|
self.assertRaises(TypeError, linkat,
|
|
"not_int", None, "not_int", None, 0)
|
|
self.assertFalse(_mock_linkat.called)
|