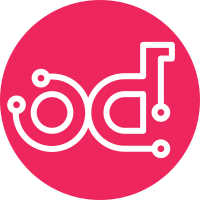
1.What is the problem: Currently Admin-API is to manage pod and pod-binding, the Admin-API access is hard coded, and only admin role is allowed. OpenStack usually use policy.json based authorization to control the API-request. Policy feature is missing in the Tricircle. 2.What's need to be fixed: Remove hard coded Admin-API request authorization, use policy instead. For Nova API-GW and Cinder API-GW, the API access control should be done at bottom OpenStack as far as possible if the API request will be forwarded to bottom OpenStack directly for further processing; only these APIs which only interact with database for example flavor and volume type, because these APIs processing will be terminated at the Tricircle layer, so policy control should be done in Nova API-GW or Cinder API-GW. No work needs to do in Tricircle Neutron Plugin for Neutron API server is there, Neutron API server will be responsible for policy control. 3.What is the purpose of this patch set: In this patch, default policy option and rule, and policy control in Admin-API were added. Using the default option and value to generate the policy.json will be implemented in next patch. No policy.json is mandatory required after this patch is merged, if no policy.json is configured or provided, the policy control will use the default rule automatically. Change-Id: Ifb6137b20f56e9f9a70d339fd357ee480fa3ce2e Signed-off-by: joehuang <joehuang@huawei.com>
83 lines
2.3 KiB
Python
83 lines
2.3 KiB
Python
# Copyright 2015 Huawei Technologies Co., Ltd.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
Routines for configuring tricircle, largely copy from Neutron
|
|
"""
|
|
import sys
|
|
|
|
from oslo_config import cfg
|
|
import oslo_log.log as logging
|
|
from oslo_policy import opts as policy_opts
|
|
|
|
from tricircle.common.i18n import _LI
|
|
|
|
from tricircle.common import policy
|
|
from tricircle.common import rpc
|
|
from tricircle.common import version
|
|
|
|
|
|
logging.register_options(cfg.CONF)
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
policy_opts.set_defaults(cfg.CONF, 'policy.json')
|
|
|
|
|
|
def init(opts, args, **kwargs):
|
|
# Register the configuration options
|
|
cfg.CONF.register_opts(opts)
|
|
|
|
cfg.CONF(args=args, project='tricircle',
|
|
version=version.version_info,
|
|
**kwargs)
|
|
|
|
_setup_logging()
|
|
_setup_policy()
|
|
|
|
rpc.init(cfg.CONF)
|
|
|
|
|
|
def _setup_logging():
|
|
"""Sets up the logging options for a log with supplied name."""
|
|
product_name = "tricircle"
|
|
logging.setup(cfg.CONF, product_name)
|
|
LOG.info(_LI("Logging enabled!"))
|
|
LOG.info(_LI("%(prog)s version %(version)s"),
|
|
{'prog': sys.argv[0],
|
|
'version': version.version_info})
|
|
LOG.debug("command line: %s", " ".join(sys.argv))
|
|
|
|
|
|
def _setup_policy():
|
|
|
|
# if there is valid policy file, use policy file by oslo_policy
|
|
# otherwise, use the default policy value in policy.py
|
|
policy_file = cfg.CONF.oslo_policy.policy_file
|
|
if policy_file and cfg.CONF.find_file(policy_file):
|
|
# just return here, oslo_policy lib will use policy file by itself
|
|
return
|
|
|
|
policy.populate_default_rules()
|
|
|
|
|
|
def reset_service():
|
|
# Reset worker in case SIGHUP is called.
|
|
# Note that this is called only in case a service is running in
|
|
# daemon mode.
|
|
_setup_logging()
|
|
|
|
policy.reset()
|
|
_setup_policy()
|