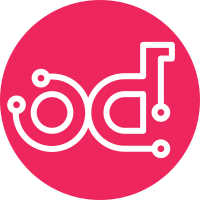
Also change parameters to what they should be according to podman specs. Add defaults for booleans in description. Improve module docs and other stuff. Change-Id: Id6e85099d6867eb873aa3bcefc1148e081812f68
352 lines
12 KiB
YAML
352 lines
12 KiB
YAML
---
|
|
- name: Test podman_image
|
|
when:
|
|
- ansible_facts.virtualization_type != 'docker'
|
|
- ansible_facts.distribution in ['RedHat', 'Fedora', 'CentOS']
|
|
block:
|
|
- name: Delete all container leftovers from tests
|
|
podman_container:
|
|
name: "{{ item }}"
|
|
state: absent
|
|
loop:
|
|
- "alpine:3.7"
|
|
- "container"
|
|
- "container2"
|
|
|
|
- name: Test no image with default action
|
|
podman_container:
|
|
name: container
|
|
ignore_errors: true
|
|
register: no_image
|
|
|
|
- name: Test no image with state 'started'
|
|
podman_container:
|
|
name: container
|
|
state: started
|
|
ignore_errors: true
|
|
register: no_image1
|
|
|
|
- name: Test no image with state 'present'
|
|
podman_container:
|
|
name: container
|
|
state: present
|
|
ignore_errors: true
|
|
register: no_image2
|
|
|
|
- name: Check no image
|
|
assert:
|
|
that:
|
|
- no_image is failed
|
|
- no_image1 is failed
|
|
- no_image2 is failed
|
|
- no_image.msg == "State 'started' required image to be configured!"
|
|
- no_image1.msg == "State 'started' required image to be configured!"
|
|
- no_image2.msg == "State 'present' required image to be configured!"
|
|
fail_msg: No image test failed!
|
|
success_msg: No image test passed!
|
|
|
|
- name: Ensure image doesn't exist
|
|
podman_image:
|
|
name: alpine:3.7
|
|
state: absent
|
|
|
|
- name: Check pulling image
|
|
podman_container:
|
|
name: container
|
|
image: alpine:3.7
|
|
state: present
|
|
command: sleep 1d
|
|
register: image
|
|
|
|
- name: Check using already pulled image
|
|
podman_container:
|
|
name: container2
|
|
image: alpine:3.7
|
|
state: present
|
|
command: sleep 1d
|
|
register: image2
|
|
|
|
- name: Check output is correct
|
|
assert:
|
|
that:
|
|
- image is changed
|
|
- image.ansible_facts is defined
|
|
- image.ansible_facts.podman_container is defined
|
|
- image.ansible_facts.podman_container['State']['Running']
|
|
- image.container is defined
|
|
- image.container['State']['Running']
|
|
- "'pulled image alpine:3.7' in image.actions"
|
|
- "'started container' in image.actions"
|
|
- image2 is changed
|
|
- image2.ansible_facts is defined
|
|
- image2.ansible_facts.podman_container is defined
|
|
- image2.ansible_facts.podman_container['State']['Running']
|
|
- image2.container is defined
|
|
- image2.container['State']['Running']
|
|
- "'pulled image alpine:3.7' not in image2.actions"
|
|
- "'started container2' in image2.actions"
|
|
fail_msg: Pulling image test failed!
|
|
success_msg: Pulling image test passed!
|
|
|
|
- name: Check failed image pull
|
|
podman_container:
|
|
name: container
|
|
image: ineverneverneverexist
|
|
state: present
|
|
command: sleep 1d
|
|
register: imagefail
|
|
ignore_errors: true
|
|
|
|
- name: Check output is correct
|
|
assert:
|
|
that:
|
|
- imagefail is failed
|
|
- imagefail.msg == "Can't pull image ineverneverneverexist"
|
|
|
|
|
|
- name: Force container recreate
|
|
podman_container:
|
|
name: container
|
|
image: alpine
|
|
state: present
|
|
command: sleep 1d
|
|
recreate: true
|
|
register: recreated
|
|
|
|
- name: Check output is correct
|
|
assert:
|
|
that:
|
|
- recreated is changed
|
|
- recreated.ansible_facts is defined
|
|
- recreated.ansible_facts.podman_container is defined
|
|
- recreated.ansible_facts.podman_container['State']['Running']
|
|
- "'recreated container' in recreated.actions"
|
|
fail_msg: Force recreate test failed!
|
|
success_msg: Force recreate test passed!
|
|
|
|
- name: Stop container
|
|
podman_container:
|
|
name: container
|
|
state: stopped
|
|
register: stopped
|
|
|
|
- name: Stop the same container again (idempotency)
|
|
podman_container:
|
|
name: container
|
|
state: stopped
|
|
register: stopped_again
|
|
|
|
- name: Check output is correct
|
|
assert:
|
|
that:
|
|
- stopped is changed
|
|
- stopped.ansible_facts is defined
|
|
- stopped.ansible_facts.podman_container is defined
|
|
- not stopped.ansible_facts.podman_container['State']['Running']
|
|
- "'stopped container' in stopped.actions"
|
|
- stopped_again is not changed
|
|
- stopped_again.ansible_facts is defined
|
|
- stopped_again.ansible_facts.podman_container is defined
|
|
- not stopped_again.ansible_facts.podman_container['State']['Running']
|
|
- stopped_again.actions == []
|
|
fail_msg: Stopping container test failed!
|
|
success_msg: Stopping container test passed!
|
|
|
|
- name: Delete stopped container
|
|
podman_container:
|
|
name: container
|
|
state: absent
|
|
register: deleted
|
|
|
|
- name: Delete again container (idempotency)
|
|
podman_container:
|
|
name: container
|
|
state: absent
|
|
register: deleted_again
|
|
|
|
- name: Check output is correct
|
|
assert:
|
|
that:
|
|
- deleted is changed
|
|
- deleted.ansible_facts is defined
|
|
- deleted.ansible_facts.podman_container is defined
|
|
- deleted.ansible_facts.podman_container == {}
|
|
- "'deleted container' in deleted.actions"
|
|
- deleted_again is not changed
|
|
- deleted_again.ansible_facts is defined
|
|
- deleted_again.ansible_facts.podman_container is defined
|
|
- deleted_again.ansible_facts.podman_container == {}
|
|
- deleted_again.actions == []
|
|
fail_msg: Deleting stopped container test failed!
|
|
success_msg: Deleting stopped container test passed!
|
|
|
|
- name: Create container, but don't run
|
|
podman_container:
|
|
name: container
|
|
image: alpine:3.7
|
|
state: stopped
|
|
command: sleep 1d
|
|
register: created
|
|
|
|
- name: Check output is correct
|
|
assert:
|
|
that:
|
|
- created is changed
|
|
- created.ansible_facts is defined
|
|
- created.ansible_facts.podman_container is defined
|
|
- created.ansible_facts.podman_container != {}
|
|
- not created.ansible_facts.podman_container['State']['Running']
|
|
- "'created container' in created.actions"
|
|
fail_msg: "Creating stopped container test failed!"
|
|
success_msg: "Creating stopped container test passed!"
|
|
|
|
- name: Delete created container
|
|
podman_container:
|
|
name: container
|
|
state: absent
|
|
|
|
- name: Start container that was deleted
|
|
podman_container:
|
|
name: container
|
|
image: alpine:3.7
|
|
state: started
|
|
command: sleep 1d
|
|
register: started
|
|
|
|
- name: Check output is correct
|
|
assert:
|
|
that:
|
|
- started is changed
|
|
- started.ansible_facts is defined
|
|
- started.ansible_facts.podman_container is defined
|
|
- started.ansible_facts.podman_container['State']['Running']
|
|
- started.container is defined
|
|
- started.container['State']['Running']
|
|
- "'pulled image alpine:3.7' not in started.actions"
|
|
|
|
- name: Delete started container
|
|
podman_container:
|
|
name: container
|
|
state: absent
|
|
register: deleted
|
|
|
|
- name: Delete again container (idempotency)
|
|
podman_container:
|
|
name: container
|
|
state: absent
|
|
register: deleted_again
|
|
|
|
- name: Check output is correct
|
|
assert:
|
|
that:
|
|
- deleted is changed
|
|
- deleted.ansible_facts is defined
|
|
- deleted.ansible_facts.podman_container is defined
|
|
- deleted.ansible_facts.podman_container == {}
|
|
- "'deleted container' in deleted.actions"
|
|
- deleted_again is not changed
|
|
- deleted_again.ansible_facts is defined
|
|
- deleted_again.ansible_facts.podman_container is defined
|
|
- deleted_again.ansible_facts.podman_container == {}
|
|
- deleted_again.actions == []
|
|
fail_msg: Deleting started container test failed!
|
|
success_msg: Deleting started container test passed!
|
|
|
|
- name: Recreate container with parameters
|
|
podman_container:
|
|
name: container
|
|
image: alpine:3.7
|
|
state: started
|
|
command: sleep 1d
|
|
recreate: true
|
|
etc_hosts:
|
|
host1: 127.0.0.1
|
|
host2: 127.0.0.1
|
|
annotation:
|
|
this: "annotation_value"
|
|
dns:
|
|
- 1.1.1.1
|
|
- 8.8.4.4
|
|
dns_search: example.com
|
|
cap_add:
|
|
- SYS_TIME
|
|
- NET_ADMIN
|
|
publish:
|
|
- "9000:80"
|
|
- "9001:8000"
|
|
workdir: "/bin"
|
|
env:
|
|
FOO: bar
|
|
BAR: foo
|
|
TEST: 1
|
|
BOOL: false
|
|
group_add: "somegroup"
|
|
label:
|
|
somelabel: labelvalue
|
|
otheralbe: othervalue
|
|
volumes:
|
|
- /tmp:/data
|
|
register: test
|
|
|
|
- name: Check output is correct
|
|
assert:
|
|
that:
|
|
- test is changed
|
|
- test.ansible_facts is defined
|
|
- test.ansible_facts.podman_container is defined
|
|
- test.ansible_facts.podman_container != {}
|
|
- test.ansible_facts.podman_container['State']['Running']
|
|
# test capabilities
|
|
- "'CAP_SYS_TIME' in test.ansible_facts.podman_container['BoundingCaps']"
|
|
- "'CAP_NET_ADMIN' in test.ansible_facts.podman_container['BoundingCaps']"
|
|
# test annotations
|
|
- test.ansible_facts.podman_container['Config']['Annotations']['this'] is defined
|
|
- test.ansible_facts.podman_container['Config']['Annotations']['this'] == "annotation_value"
|
|
# test DNS
|
|
- >-
|
|
(test.ansible_facts.podman_container['HostConfig']['Dns'] is defined and
|
|
test.ansible_facts.podman_container['HostConfig']['Dns'] == ['1.1.1.1', '8.8.4.4']) or
|
|
(test.ansible_facts.podman_container['HostConfig']['DNS'] is defined and
|
|
test.ansible_facts.podman_container['HostConfig']['DNS'] == ['1.1.1.1', '8.8.4.4'])
|
|
# test ports
|
|
- test.ansible_facts.podman_container['NetworkSettings']['Ports']|length == 2
|
|
# test working dir
|
|
- test.ansible_facts.podman_container['Config']['WorkingDir'] == "/bin"
|
|
# test dns search
|
|
- >-
|
|
(test.ansible_facts.podman_container['HostConfig']['DnsSearch'] is defined and
|
|
test.ansible_facts.podman_container['HostConfig']['DnsSearch'] == ['example.com']) or
|
|
(test.ansible_facts.podman_container['HostConfig']['DNSSearch'] is defined and
|
|
test.ansible_facts.podman_container['HostConfig']['DNSSearch'] == ['example.com'])
|
|
# test environment variables
|
|
- "'FOO=bar' in test.ansible_facts.podman_container['Config']['Env']"
|
|
- "'BAR=foo' in test.ansible_facts.podman_container['Config']['Env']"
|
|
- "'TEST=1' in test.ansible_facts.podman_container['Config']['Env']"
|
|
- "'BOOL=False' in test.ansible_facts.podman_container['Config']['Env']"
|
|
# test labels
|
|
- test.ansible_facts.podman_container['Config']['Labels'] | length == 2
|
|
- test.ansible_facts.podman_container['Config']['Labels']['somelabel'] == "labelvalue"
|
|
- test.ansible_facts.podman_container['Config']['Labels']['otheralbe'] == "othervalue"
|
|
# test mounts
|
|
- >-
|
|
(test.ansible_facts.podman_container['Mounts'][0]['Destination'] is defined and
|
|
'/data' in test.ansible_facts.podman_container['Mounts'] | map(attribute='Destination') | list) or
|
|
(test.ansible_facts.podman_container['Mounts'][0]['destination'] is defined and
|
|
'/data' in test.ansible_facts.podman_container['Mounts'] | map(attribute='destination') | list)
|
|
- >-
|
|
(test.ansible_facts.podman_container['Mounts'][0]['Source'] is defined and
|
|
'/tmp' in test.ansible_facts.podman_container['Mounts'] | map(attribute='Source') | list) or
|
|
(test.ansible_facts.podman_container['Mounts'][0]['source'] is defined and
|
|
'/tmp' in test.ansible_facts.podman_container['Mounts'] | map(attribute='source') | list)
|
|
fail_msg: Parameters container test failed!
|
|
success_msg: Parameters container test passed!
|
|
always:
|
|
- name: Delete all container leftovers from tests
|
|
podman_container:
|
|
name: "{{ item }}"
|
|
state: absent
|
|
loop:
|
|
- "alpine:3.7"
|
|
- "container"
|
|
- "container2"
|