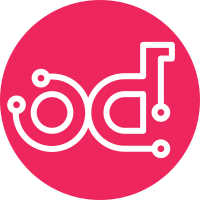
The missing quotes didn't trigger anything since "vars" is representing
the vars() method...
Adding the missing quotes allows to actually get the injected host
variables in the inventory, among them the well needed "container_cli"
for the validations.
This patch also makes a huge update in the different inline dict, in
order to make them more human readable. This should help for further
editions.
Closes-Bug: #1889635
Change-Id: I7418ea45d89dd0da7ac39193200f47563acab4cb
(cherry picked from commit e2613f0317
)
147 lines
5.0 KiB
Python
147 lines
5.0 KiB
Python
# -*- coding: utf-8 -*-
|
|
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import collections
|
|
import fixtures
|
|
import os
|
|
import yaml
|
|
|
|
from mock import MagicMock
|
|
from tripleo_common.tests import base
|
|
from tripleo_common.inventories import TripleoInventories
|
|
|
|
|
|
class _TestInventoriesBase(base.TestCase):
|
|
def setUp(self):
|
|
super(_TestInventoriesBase, self).setUp()
|
|
self.read_inventory_data()
|
|
|
|
def read_inventory_data(self):
|
|
inventory_data = collections.OrderedDict()
|
|
inventory_dir = os.path.join(
|
|
os.path.dirname(__file__), 'inventory_data'
|
|
)
|
|
for datafile in (
|
|
'cell1_dynamic.json',
|
|
'cell1_static.yaml',
|
|
'overcloud_dynamic.json',
|
|
'overcloud_static.yaml',
|
|
'merged_dynamic.json',
|
|
'merged_static.yaml',
|
|
'single_dynamic.json',
|
|
'single_static.yaml',
|
|
|
|
):
|
|
name = os.path.basename(datafile).split('.')[0]
|
|
path = os.path.join(inventory_dir, datafile)
|
|
with open(path, 'r') as data:
|
|
inventory_data[name] = yaml.safe_load(data)
|
|
self.inventory_data = inventory_data
|
|
|
|
|
|
class TestInventoriesStatic(_TestInventoriesBase):
|
|
def setUp(self):
|
|
super(TestInventoriesStatic, self).setUp()
|
|
mock_inv_overcloud = MagicMock()
|
|
mock_inv_cell1 = MagicMock()
|
|
mock_inv_overcloud.list.return_value = self.inventory_data[
|
|
'overcloud_static'
|
|
]
|
|
mock_inv_cell1.list.return_value = self.inventory_data[
|
|
'cell1_static'
|
|
]
|
|
stack_to_inv_obj_map = {
|
|
'overcloud': mock_inv_overcloud,
|
|
'cell1': mock_inv_cell1
|
|
}
|
|
self.inventories = TripleoInventories(stack_to_inv_obj_map)
|
|
|
|
def test_merge(self):
|
|
actual = dict(self.inventories._merge(dynamic=False))
|
|
expected = self.inventory_data['merged_static']
|
|
self.assertEqual(expected, actual)
|
|
|
|
def test_inventory_write_static(self):
|
|
tmp_dir = self.useFixture(fixtures.TempDir()).path
|
|
inv_path = os.path.join(tmp_dir, "inventory.yaml")
|
|
self.inventories.write_static_inventory(inv_path)
|
|
expected = self.inventory_data['merged_static']
|
|
with open(inv_path, 'r') as f:
|
|
loaded_inv = collections.OrderedDict(yaml.safe_load(f))
|
|
self.assertEqual(expected, loaded_inv)
|
|
|
|
|
|
class TestInventoriesDynamic(_TestInventoriesBase):
|
|
def setUp(self):
|
|
super(TestInventoriesDynamic, self).setUp()
|
|
mock_inv_overcloud = MagicMock()
|
|
mock_inv_cell1 = MagicMock()
|
|
mock_inv_overcloud.list.return_value = self.inventory_data[
|
|
'overcloud_dynamic'
|
|
]
|
|
mock_inv_cell1.list.return_value = self.inventory_data[
|
|
'cell1_dynamic'
|
|
]
|
|
stack_to_inv_obj_map = {
|
|
'overcloud': mock_inv_overcloud,
|
|
'cell1': mock_inv_cell1
|
|
}
|
|
self.inventories = TripleoInventories(stack_to_inv_obj_map)
|
|
|
|
def test_merge(self):
|
|
actual = dict(self.inventories._merge())
|
|
expected = dict(self.inventory_data['merged_dynamic'])
|
|
self.assertEqual(expected, actual)
|
|
|
|
def test_list(self):
|
|
actual = self.inventories.list()
|
|
expected = self.inventory_data['merged_dynamic']
|
|
self.assertEqual(expected, actual)
|
|
|
|
|
|
class TestInventorySingleStatic(_TestInventoriesBase):
|
|
def setUp(self):
|
|
super(TestInventorySingleStatic, self).setUp()
|
|
mock_inv_overcloud = MagicMock()
|
|
mock_inv_overcloud.list.return_value = self.inventory_data[
|
|
'overcloud_static'
|
|
]
|
|
stack_to_inv_obj_map = {
|
|
'overcloud': mock_inv_overcloud
|
|
}
|
|
self.inventories = TripleoInventories(stack_to_inv_obj_map)
|
|
|
|
def test_list(self):
|
|
actual = self.inventories.list()
|
|
expected = self.inventory_data['single_static']
|
|
self.assertEqual(expected, actual)
|
|
|
|
|
|
class TestInventorySingleDynamic(_TestInventoriesBase):
|
|
def setUp(self):
|
|
super(TestInventorySingleDynamic, self).setUp()
|
|
mock_inv_overcloud = MagicMock()
|
|
mock_inv_overcloud.list.return_value = self.inventory_data[
|
|
'overcloud_dynamic'
|
|
]
|
|
stack_to_inv_obj_map = {
|
|
'overcloud': mock_inv_overcloud
|
|
}
|
|
self.inventories = TripleoInventories(stack_to_inv_obj_map)
|
|
|
|
def test_list(self):
|
|
actual = self.inventories.list()
|
|
expected = self.inventory_data['single_dynamic']
|
|
self.assertEqual(expected, actual)
|