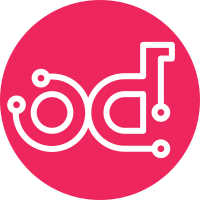
This change will drop icons on buttons where they aren't needed. This will simplify buttons and continue to push for consistency where buttons don't need icons. The buttons dropping icons are: -Import Plan button on Plans page -Validate and Deploy button on Plan Details page -Deploy button on Deploy Confirmation modal -Register Nodes button on Plan Details page -Register Nodes button on Nodes page -Add New button on Register Nodes modal -Upload From File button on Register Nodes modal Change-Id: Ibf9a025f4045f25416a529e281637b6c3292d4cf Closes-Bug: #1753530
124 lines
3.8 KiB
JavaScript
124 lines
3.8 KiB
JavaScript
/**
|
|
* Copyright 2017 Red Hat Inc.
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
* not use this file except in compliance with the License. You may obtain
|
|
* a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
* License for the specific language governing permissions and limitations
|
|
* under the License.
|
|
*/
|
|
|
|
import { defineMessages, injectIntl, FormattedMessage } from 'react-intl';
|
|
import ImmutablePropTypes from 'react-immutable-proptypes';
|
|
import PropTypes from 'prop-types';
|
|
import React from 'react';
|
|
|
|
import DeploymentSuccess from './DeploymentSuccess';
|
|
import DeploymentFailure from './DeploymentFailure';
|
|
import DeploymentProgress from './DeploymentProgress';
|
|
import Link from '../ui/Link';
|
|
import { InlineLoader, Loader } from '../ui/Loader';
|
|
import { stackStates } from '../../constants/StacksConstants';
|
|
|
|
const messages = defineMessages({
|
|
validateAndDeploy: {
|
|
id: 'DeployStep.validateAndDeploy',
|
|
defaultMessage: 'Validate and Deploy'
|
|
},
|
|
requestingDeploy: {
|
|
id: 'DeployStep.requestingDeploy',
|
|
defaultMessage: 'Requesting a deploy...'
|
|
}
|
|
});
|
|
|
|
export const DeployStep = ({
|
|
currentPlan,
|
|
currentStack,
|
|
currentStackResources,
|
|
currentStackDeploymentProgress,
|
|
deleteStack,
|
|
deployPlan,
|
|
fetchStackResource,
|
|
fetchStackEnvironment,
|
|
intl,
|
|
isRequestingStackDelete,
|
|
overcloudInfo,
|
|
stacksLoaded
|
|
}) => {
|
|
if (
|
|
!currentStack ||
|
|
currentStack.stack_status === stackStates.DELETE_COMPLETE
|
|
) {
|
|
return (
|
|
<Loader loaded={stacksLoaded}>
|
|
<Link
|
|
className="link btn btn-primary btn-lg"
|
|
disabled={currentPlan.isRequestingPlanDeploy}
|
|
to={`/plans/${currentPlan.name}/deployment-detail`}
|
|
>
|
|
<InlineLoader
|
|
loaded={!currentPlan.isRequestingPlanDeploy}
|
|
content={intl.formatMessage(messages.requestingDeploy)}
|
|
>
|
|
<FormattedMessage {...messages.validateAndDeploy} />
|
|
</InlineLoader>
|
|
</Link>
|
|
</Loader>
|
|
);
|
|
} else if (currentStack.stack_status.match(/PROGRESS/)) {
|
|
return (
|
|
<DeploymentProgress
|
|
currentPlanName={currentPlan.name}
|
|
stack={currentStack}
|
|
isRequestingStackDelete={isRequestingStackDelete}
|
|
deleteStack={deleteStack}
|
|
deploymentProgress={currentStackDeploymentProgress}
|
|
/>
|
|
);
|
|
} else if (currentStack.stack_status.match(/COMPLETE/)) {
|
|
return (
|
|
<DeploymentSuccess
|
|
stack={currentStack}
|
|
stackResources={currentStackResources}
|
|
isRequestingStackDelete={isRequestingStackDelete}
|
|
overcloudInfo={overcloudInfo}
|
|
deleteStack={deleteStack}
|
|
fetchStackResource={fetchStackResource}
|
|
fetchStackEnvironment={fetchStackEnvironment}
|
|
/>
|
|
);
|
|
} else {
|
|
return (
|
|
<DeploymentFailure
|
|
currentPlanName={currentPlan.name}
|
|
deleteStack={deleteStack}
|
|
isRequestingStackDelete={isRequestingStackDelete}
|
|
stack={currentStack}
|
|
/>
|
|
);
|
|
}
|
|
};
|
|
|
|
DeployStep.propTypes = {
|
|
currentPlan: ImmutablePropTypes.record.isRequired,
|
|
currentStack: ImmutablePropTypes.record,
|
|
currentStackDeploymentProgress: PropTypes.number.isRequired,
|
|
currentStackResources: ImmutablePropTypes.map,
|
|
deleteStack: PropTypes.func.isRequired,
|
|
deployPlan: PropTypes.func.isRequired,
|
|
fetchStackEnvironment: PropTypes.func.isRequired,
|
|
fetchStackResource: PropTypes.func.isRequired,
|
|
intl: PropTypes.object,
|
|
isRequestingStackDelete: PropTypes.bool.isRequired,
|
|
overcloudInfo: ImmutablePropTypes.map.isRequired,
|
|
stacksLoaded: PropTypes.bool.isRequired
|
|
};
|
|
|
|
export default injectIntl(DeployStep);
|