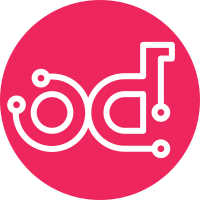
Adds support for root-enable for Vertica clusters, with optional user-provided passwords. In order to support root-enable for clusters, a new route was added. A new VerticaRootController extends the features that the RootController provides for clusters. This datastore specific controller is added to the subfolder vertica in extensions. Added unit tests for new Vertica functionalities. Refactoring: 1. Made this patch dependant on pxc patch, in order to be able to address these changes for pxc clustering. Also added rootController entry to pxc's config 2. Moved all the RootEnable code inside trove/extensions/common. Since there was nothing specific to mysql in that code. 3. Changed the cfg.py to now include a default RootController for all the datastores. Because without this patch all the datastores were going through the same old mysql root controller anyways, so no need to change the bahavior of the other datastores. Which ever datastores don't provide root implementation they will raise appropriate exceptions anyways. 4. If root_password is not passed then calling enable_root, if the password is supplied then calling enable_root_with_password. That way the datastores which provide enable_root implementation but not enable_root_with_password can be handled correctly, appropriate exceptions are raised to indicate to user that supplied password will not be honored. 5. If root-enable is called on clusters, then default root controller implementation raises exception, as other than vertica nobody provides the implementation for it as of now. For vertica, this is processed correctly. Change-Id: I28209ab562b26f45742b5db65c5cc057e07f7f51 Implements blueprint: vertica-cluster-user-features Depends-On: I3e23c87c0e48a7f910e54207116fc75b5ce0ad0c
75 lines
1.9 KiB
Python
75 lines
1.9 KiB
Python
# Copyright 2011 OpenStack Foundation
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
|
|
class UserView(object):
|
|
|
|
def __init__(self, user):
|
|
self.user = user
|
|
|
|
def data(self):
|
|
user_dict = {
|
|
"name": self.user.name,
|
|
"host": self.user.host,
|
|
"databases": self.user.databases
|
|
}
|
|
return {"user": user_dict}
|
|
|
|
|
|
class UsersView(object):
|
|
|
|
def __init__(self, users):
|
|
self.users = users
|
|
|
|
def data(self):
|
|
userlist = [{"name": user.name,
|
|
"host": user.host,
|
|
"databases": user.databases}
|
|
for user in self.users]
|
|
|
|
return {"users": userlist}
|
|
|
|
|
|
class UserAccessView(object):
|
|
def __init__(self, databases):
|
|
self.databases = databases
|
|
|
|
def data(self):
|
|
dbs = [{"name": db.name} for db in self.databases]
|
|
return {"databases": dbs}
|
|
|
|
|
|
class SchemaView(object):
|
|
|
|
def __init__(self, schema):
|
|
self.schema = schema
|
|
|
|
def data(self):
|
|
return {"name": self.schema.name}
|
|
|
|
|
|
class SchemasView(object):
|
|
|
|
def __init__(self, schemas):
|
|
self.schemas = schemas
|
|
|
|
def data(self):
|
|
data = []
|
|
# These are model instances
|
|
for schema in self.schemas:
|
|
data.append(SchemaView(schema).data())
|
|
|
|
return {"databases": data}
|