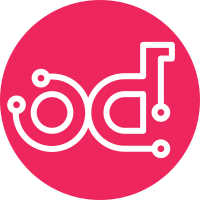
* Changed reddwarf-server to load the paste config first so the config file valeus would be read during start up. * Made the Config class update the dictionary values instead of replace them all. * Added a test conf file for running the server locally. * Added a fake version of Nova and the Guest API. * Changed Guest API to not require every single method to pass context and ID. This also makes it easier to fake. * Changed some instances of uuid to id. * Added "BUILD" server status to list of statuses which should be returned instantly in Instance status code.
74 lines
2.3 KiB
Python
Executable File
74 lines
2.3 KiB
Python
Executable File
#!/usr/bin/env python
|
|
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright 2011 OpenStack LLC.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import gettext
|
|
import optparse
|
|
import os
|
|
import sys
|
|
|
|
|
|
gettext.install('reddwarf', unicode=1)
|
|
|
|
|
|
# If ../reddwarf/__init__.py exists, add ../ to Python search path, so that
|
|
# it will override what happens to be installed in /usr/(local/)lib/python...
|
|
possible_topdir = os.path.normpath(os.path.join(os.path.abspath(sys.argv[0]),
|
|
os.pardir,
|
|
os.pardir))
|
|
if os.path.exists(os.path.join(possible_topdir, 'reddwarf', '__init__.py')):
|
|
sys.path.insert(0, possible_topdir)
|
|
|
|
from reddwarf import version
|
|
from reddwarf.common import config
|
|
from reddwarf.common import wsgi
|
|
from reddwarf.db import db_api
|
|
|
|
|
|
def create_options(parser):
|
|
"""Sets up the CLI and config-file options
|
|
|
|
:param parser: The option parser
|
|
:returns: None
|
|
|
|
"""
|
|
parser.add_option('-p', '--port', dest="port", metavar="PORT",
|
|
type=int, default=8779,
|
|
help="Port the Reddwarf API host listens on. "
|
|
"Default: %default")
|
|
config.add_common_options(parser)
|
|
config.add_log_options(parser)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
oparser = optparse.OptionParser(version="%%prog %s"
|
|
% version.version_string())
|
|
create_options(oparser)
|
|
(options, args) = config.parse_options(oparser)
|
|
try:
|
|
config.Config.load_paste_config('reddwarf', options, args)
|
|
conf, app = config.Config.load_paste_app('reddwarf', options, args)
|
|
db_api.configure_db(conf)
|
|
server = wsgi.Server()
|
|
server.start(app, options.get('port', conf['bind_port']),
|
|
conf['bind_host'])
|
|
server.wait()
|
|
except RuntimeError as error:
|
|
import traceback
|
|
print traceback.format_exc()
|
|
sys.exit("ERROR: %s" % error)
|