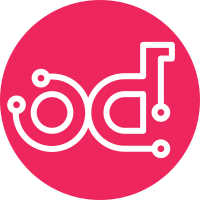
- Added migrate changes for new database artifacts. - Added models for Security Groups and Security Group Rules. - Definition and addition of Controllers for API. - Views for Security Groups and Security Group rules. - Extended taskmanager to use newly created Security Group on instance creation, and to clean it up on deletion if extension is enabled - Added new flag to conf to enable "reddwarf_security_groups_support" - Integration tests. Implements blueprint: security-groups Change-Id: I44053359c12e53a9e549bd334e628ecd249d8ba0
74 lines
2.5 KiB
Python
74 lines
2.5 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright 2013 Hewlett-Packard Development Company, L.P.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
#
|
|
|
|
from reddwarf.openstack.common import log as logging
|
|
|
|
from reddwarf.common import extensions
|
|
from reddwarf.common import wsgi
|
|
from reddwarf.common import cfg
|
|
from reddwarf.extensions.security_group import service
|
|
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
CONF = cfg.CONF
|
|
|
|
|
|
# The Extensions module from openstack common expects the classname of the
|
|
# extension to be loaded to be the exact same as the filename, except with
|
|
# a capital first letter. That's the reason this class has such a funky name.
|
|
class Security_group(extensions.ExtensionsDescriptor):
|
|
|
|
def get_name(self):
|
|
return "SecurityGroup"
|
|
|
|
def get_description(self):
|
|
return "Security Group related operations such as list \
|
|
security groups and manage security group rules."
|
|
|
|
def get_alias(self):
|
|
return "SecurityGroup"
|
|
|
|
def get_namespace(self):
|
|
return "http://TBD"
|
|
|
|
def get_updated(self):
|
|
return "2012-02-26T17:25:27-08:00"
|
|
|
|
def get_resources(self):
|
|
resources = []
|
|
serializer = wsgi.ReddwarfResponseSerializer(
|
|
body_serializers={'application/xml':
|
|
wsgi.ReddwarfXMLDictSerializer()})
|
|
|
|
if CONF.reddwarf_security_groups_support:
|
|
security_groups = extensions.ResourceExtension(
|
|
'{tenant_id}/security_groups',
|
|
service.SecurityGroupController(),
|
|
deserializer=wsgi.ReddwarfRequestDeserializer(),
|
|
serializer=serializer)
|
|
resources.append(security_groups)
|
|
|
|
security_group_rules = extensions.ResourceExtension(
|
|
'{tenant_id}/security_group_rules',
|
|
service.SecurityGroupRuleController(),
|
|
deserializer=wsgi.ReddwarfRequestDeserializer(),
|
|
serializer=serializer)
|
|
resources.append(security_group_rules)
|
|
|
|
return resources
|