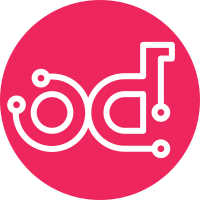
added some helper code to make sure the mysql service is up add a config value to turn on volume support moving around the lines to be dependent on volume support trying to stop volume support fixes with the merge imported and raised exceptions in guest correctly fixed the config get values as boolean and not just the string deleting the serivce status entry on delete of the instance so that the guest can not update the instance to active again. made the create and update times saved correctly for an instance check if mysql is already installed in the guest clean up instance model using _(i8n) adding the populate databases back in pep8 compliance updated apt get version method moved the volumeHelper class to a new module made the start/stop mysql methods public created a method to create volume for instances and readability created a new client for volume calls added the volume and size to the create/list/show instance api calls removing the mount_point and device_path from the MysqlApp class removing code that deleted the instance status entry adding volume service to the reddwarf.conf.test file set volumes to not be disabled by default
119 lines
4.1 KiB
Python
119 lines
4.1 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright (c) 2011 OpenStack, LLC.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
Handles all processes within the Guest VM, considering it as a Platform
|
|
|
|
The :py:class:`GuestManager` class is a :py:class:`nova.manager.Manager` that
|
|
handles RPC calls relating to Platform specific operations.
|
|
|
|
"""
|
|
|
|
|
|
import functools
|
|
import logging
|
|
import traceback
|
|
|
|
from reddwarf.common import config
|
|
from reddwarf.common import exception
|
|
from reddwarf.common import utils
|
|
from reddwarf.common import service
|
|
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
CONFIG = config.Config
|
|
GUEST_SERVICES = {'mysql': 'reddwarf.guestagent.dbaas.DBaaSAgent'}
|
|
|
|
|
|
class GuestManager(service.Manager):
|
|
|
|
"""Manages the tasks within a Guest VM."""
|
|
|
|
def __init__(self, guest_drivers=None, *args, **kwargs):
|
|
service_type = CONFIG.get('service_type')
|
|
try:
|
|
service_impl = GUEST_SERVICES[service_type]
|
|
except KeyError as e:
|
|
LOG.error(_("Could not create guest, no impl for key - %s") %
|
|
service_type)
|
|
raise e
|
|
LOG.info("Create guest driver %s" % service_impl)
|
|
self.create_guest_driver(service_impl)
|
|
super(GuestManager, self).__init__(*args, **kwargs)
|
|
|
|
def create_guest_driver(self, service_impl):
|
|
guest_drivers = [service_impl,
|
|
'reddwarf.guestagent.pkg.PkgAgent']
|
|
classes = []
|
|
for guest_driver in guest_drivers:
|
|
LOG.info(guest_driver)
|
|
driver = utils.import_class(guest_driver)
|
|
classes.append(driver)
|
|
try:
|
|
cls = type("GuestDriver", tuple(set(classes)), {})
|
|
self.driver = cls()
|
|
except TypeError as te:
|
|
msg = "An issue occurred instantiating the GuestDriver as the " \
|
|
"following classes: " + str(classes) + \
|
|
" Exception=" + str(te)
|
|
raise TypeError(msg)
|
|
|
|
def init_host(self):
|
|
"""Method for any service initialization"""
|
|
pass
|
|
|
|
def periodic_tasks(self, raise_on_error=False):
|
|
"""Method for running any periodic tasks.
|
|
|
|
Right now does the status updates"""
|
|
status_method = "update_status"
|
|
try:
|
|
method = getattr(self.driver, status_method)
|
|
except AttributeError as ae:
|
|
LOG.error(_("Method %s not found for driver %s"), status_method,
|
|
self.driver)
|
|
if raise_on_error:
|
|
raise ae
|
|
try:
|
|
method()
|
|
except Exception as e:
|
|
LOG.error("Got an error during periodic tasks!")
|
|
LOG.debug(traceback.format_exc())
|
|
|
|
def upgrade(self, context):
|
|
"""Upgrade the guest agent and restart the agent"""
|
|
LOG.debug(_("Self upgrade of guest agent issued"))
|
|
|
|
def __getattr__(self, key):
|
|
"""Converts all method calls and direct it at the driver"""
|
|
return functools.partial(self._mapper, key)
|
|
|
|
def _mapper(self, method, context, *args, **kwargs):
|
|
""" Tries to call the respective driver method """
|
|
try:
|
|
func = getattr(self.driver, method)
|
|
except AttributeError:
|
|
LOG.error(_("Method %s not found for driver %s"), method,
|
|
self.driver)
|
|
raise exception.NotFound("Method %s is not available for the "
|
|
"chosen driver.")
|
|
try:
|
|
return func(*args, **kwargs)
|
|
except Exception as e:
|
|
LOG.error("Got an error running %s!" % method)
|
|
LOG.debug(traceback.format_exc())
|