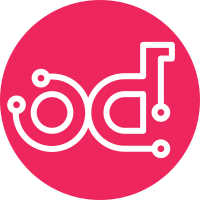
Each filecontent is stored uniquely by sha1 so multiple files can refer to the same filecontent. Let's expose the sha1 for files so we can use this client side if need be. Change-Id: Iddd4701f8b9cc5f4b579854f495fffc6efbf04ac
104 lines
4.4 KiB
Python
104 lines
4.4 KiB
Python
# Copyright (c) 2018 Red Hat, Inc.
|
|
#
|
|
# This file is part of ARA Records Ansible.
|
|
#
|
|
# ARA is free software: you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License as published by
|
|
# the Free Software Foundation, either version 3 of the License, or
|
|
# (at your option) any later version.
|
|
#
|
|
# ARA is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with ARA. If not, see <http://www.gnu.org/licenses/>.
|
|
|
|
from rest_framework.test import APITestCase
|
|
|
|
from ara.api import models, serializers
|
|
from ara.api.tests import factories, utils
|
|
|
|
|
|
class FileTestCase(APITestCase):
|
|
def test_file_factory(self):
|
|
file_content = factories.FileContentFactory()
|
|
file = factories.FileFactory(path="/path/playbook.yml", content=file_content)
|
|
self.assertEqual(file.path, "/path/playbook.yml")
|
|
self.assertEqual(file.content.sha1, file_content.sha1)
|
|
|
|
def test_file_serializer(self):
|
|
serializer = serializers.FileSerializer(data={"path": "/path/playbook.yml", "content": factories.FILE_CONTENTS})
|
|
serializer.is_valid()
|
|
file = serializer.save()
|
|
file.refresh_from_db()
|
|
self.assertEqual(file.content.sha1, utils.sha1(factories.FILE_CONTENTS))
|
|
|
|
def test_create_file_with_same_content_create_only_one_file_content(self):
|
|
serializer = serializers.FileSerializer(
|
|
data={"path": "/path/1/playbook.yml", "content": factories.FILE_CONTENTS}
|
|
)
|
|
serializer.is_valid()
|
|
file_content = serializer.save()
|
|
file_content.refresh_from_db()
|
|
|
|
serializer2 = serializers.FileSerializer(
|
|
data={"path": "/path/2/playbook.yml", "content": factories.FILE_CONTENTS}
|
|
)
|
|
serializer2.is_valid()
|
|
file_content = serializer2.save()
|
|
file_content.refresh_from_db()
|
|
|
|
self.assertEqual(2, models.File.objects.all().count())
|
|
self.assertEqual(1, models.FileContent.objects.all().count())
|
|
|
|
def test_create_file(self):
|
|
self.assertEqual(0, models.File.objects.count())
|
|
request = self.client.post("/api/v1/files", {"path": "/path/playbook.yml", "content": factories.FILE_CONTENTS})
|
|
self.assertEqual(201, request.status_code)
|
|
self.assertEqual(1, models.File.objects.count())
|
|
|
|
def test_get_no_files(self):
|
|
request = self.client.get("/api/v1/files")
|
|
self.assertEqual(0, len(request.data["results"]))
|
|
|
|
def test_get_files(self):
|
|
file = factories.FileFactory()
|
|
request = self.client.get("/api/v1/files")
|
|
self.assertEqual(1, len(request.data["results"]))
|
|
self.assertEqual(file.path, request.data["results"][0]["path"])
|
|
|
|
def test_get_file(self):
|
|
file = factories.FileFactory()
|
|
request = self.client.get("/api/v1/files/%s" % file.id)
|
|
self.assertEqual(file.path, request.data["path"])
|
|
self.assertEqual(file.content.sha1, request.data["sha1"])
|
|
|
|
def test_update_file(self):
|
|
file = factories.FileFactory()
|
|
old_sha1 = file.content.sha1
|
|
self.assertNotEqual("/path/new_playbook.yml", file.path)
|
|
request = self.client.put(
|
|
"/api/v1/files/%s" % file.id, {"path": "/path/new_playbook.yml", "content": "# playbook"}
|
|
)
|
|
self.assertEqual(200, request.status_code)
|
|
file_updated = models.File.objects.get(id=file.id)
|
|
self.assertEqual("/path/new_playbook.yml", file_updated.path)
|
|
self.assertNotEqual(old_sha1, file_updated.content.sha1)
|
|
|
|
def test_partial_update_file(self):
|
|
file = factories.FileFactory()
|
|
self.assertNotEqual("/path/new_playbook.yml", file.path)
|
|
request = self.client.patch("/api/v1/files/%s" % file.id, {"path": "/path/new_playbook.yml"})
|
|
self.assertEqual(200, request.status_code)
|
|
file_updated = models.File.objects.get(id=file.id)
|
|
self.assertEqual("/path/new_playbook.yml", file_updated.path)
|
|
|
|
def test_delete_file(self):
|
|
file = factories.FileFactory()
|
|
self.assertEqual(1, models.File.objects.all().count())
|
|
request = self.client.delete("/api/v1/files/%s" % file.id)
|
|
self.assertEqual(204, request.status_code)
|
|
self.assertEqual(0, models.File.objects.all().count())
|