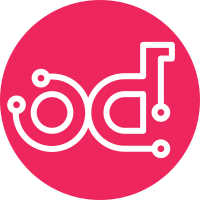
When a ceph command is executed, the exit code is always "0" regardless of whether the command was successful or not. This happens because in the CLI, the command that is executed within the toolbox pod is not the last one, so it always returns the last command, which is always 0. So to solve this problem, the exit code of the command sent to the toolbox pod is checked and stored, and if it is non-zero, the execution is terminated there. Test Plan: - PASS: AIO-SX with rook ceph backend configured - PASS: Run 'ceph -s' command and check exit code - PASS: Run 'ceph test-123' command and check exit code Story: 2011066 Task: 51021 Change-Id: I0b4c08da57c4c54833b7d75615215b7840275ce4 Signed-off-by: Erickson Silva de Oliveira <Erickson.SilvadeOliveira@windriver.com>
143 lines
3.7 KiB
Bash
143 lines
3.7 KiB
Bash
#!/bin/bash
|
|
|
|
# Copyright (c) 2024 Wind River Systems, Inc.
|
|
#
|
|
# SPDX-License-Identifier: Apache-2.0
|
|
#
|
|
|
|
# This script is wrapper for ceph, providing access to
|
|
# bare-metal or rook, according to defined backend.
|
|
|
|
NODE_ROOK_CONFIGURED_FLAG="/etc/platform/.node_rook_configured"
|
|
NODE_CEPH_CONFIGURED_FLAG="/etc/platform/.node_ceph_configured"
|
|
|
|
PLATFORM_CONF="/etc/platform/platform.conf"
|
|
|
|
ROOK_CEPH_NAMESPACE="rook-ceph"
|
|
ROOK_CEPH_TOOLS_NAME="rook-ceph-tools"
|
|
KUBE_CONFIG="/etc/kubernetes/admin.conf"
|
|
|
|
# Logger setup
|
|
LOG_FACILITY=user
|
|
LOG_PRIORITY=info
|
|
|
|
ARGS=("$@")
|
|
COMPLETION=false
|
|
CMD_DIR="/tmp"
|
|
|
|
function LOG {
|
|
if [ "${COMPLETION}" = false ]; then
|
|
logger -t "${0##*/}[$$]" -p ${LOG_FACILITY}.${LOG_PRIORITY} "$@"
|
|
echo "$@"
|
|
fi
|
|
}
|
|
|
|
function process_argument {
|
|
local FILENAME
|
|
local ARG=$1
|
|
FILENAME=$(basename -- "$ARG")
|
|
local REMOTE_FILE=${CMD_DIR}/${FILENAME}
|
|
ARGS="${ARGS/$ARG/$REMOTE_FILE}"
|
|
if [ "$2" = "in" ]; then
|
|
kubectl --kubeconfig ${KUBE_CONFIG} cp "${ARG}" \
|
|
-n ${ROOK_CEPH_NAMESPACE} "${ROOK_CEPH_TOOLS_POD}":"${CMD_DIR}"
|
|
elif [ "$2" = "out" ]; then
|
|
OUTPUT_REMOTE=$REMOTE_FILE
|
|
fi
|
|
}
|
|
|
|
for ((i=1;i<=$#;i++)); do
|
|
if [ "${!i}" = "-i" ] || [ "${!i}" = "--in-file" ]; then
|
|
i=$((i+1))
|
|
INPUT_ARG=${!i};
|
|
fi
|
|
|
|
if [ "${!i}" = "-o" ] || [ "${!i}" = "--out-file" ]; then
|
|
i=$((i+1))
|
|
OUTPUT_ARG=${!i};
|
|
fi
|
|
|
|
if [ "${!i}" = "-c" ] || [ "${!i}" = "--conf" ]; then
|
|
i=$((i+1))
|
|
CONF_ARG=${!i};
|
|
fi
|
|
done;
|
|
|
|
if [[ "${ARGS}" == *" " ]]; then
|
|
COMPLETION=true
|
|
fi
|
|
|
|
if [ -f "${NODE_ROOK_CONFIGURED_FLAG}" ]; then
|
|
. $PLATFORM_CONF
|
|
if [[ $nodetype != "controller" ]]; then
|
|
LOG "Rook CLI access is only available on control plane hosts."
|
|
exit
|
|
fi
|
|
|
|
# Get rook-ceph-tools pod state
|
|
ROOK_CEPH_TOOLS_POD=$(kubectl --kubeconfig ${KUBE_CONFIG} \
|
|
get pods -n ${ROOK_CEPH_NAMESPACE} \
|
|
--selector=app=${ROOK_CEPH_TOOLS_NAME} \
|
|
--field-selector=status.phase==Running \
|
|
-o jsonpath="{.items[0].metadata.name}" 2>/dev/null)
|
|
RETURN_CODE=$?
|
|
|
|
if [ ${RETURN_CODE} -ne 0 ]; then
|
|
LOG "The rook toolbox is not running."
|
|
exit ${RETURN_CODE}
|
|
fi
|
|
|
|
if [ -z "${ARGS}" ]; then
|
|
# Interactive Rook toolbox
|
|
kubectl --kubeconfig ${KUBE_CONFIG} \
|
|
exec -it -n ${ROOK_CEPH_NAMESPACE} \
|
|
deploy/${ROOK_CEPH_TOOLS_NAME} -- /usr/bin/ceph
|
|
else
|
|
for arg in "${ARGS[@]}"; do
|
|
_ARGS="$_ARGS '${arg}'"
|
|
done
|
|
ARGS=$_ARGS
|
|
|
|
if [[ $INPUT_ARG ]]; then
|
|
process_argument "$INPUT_ARG" "in"
|
|
fi
|
|
|
|
if [[ $CONF_ARG ]]; then
|
|
process_argument "$CONF_ARG" "in"
|
|
fi
|
|
|
|
if [[ $OUTPUT_ARG ]]; then
|
|
process_argument "$OUTPUT_ARG" "out"
|
|
fi
|
|
|
|
if [ "${COMPLETION}" = true ]; then
|
|
ARGS+=" ''"
|
|
fi
|
|
|
|
# Execute single ceph command in Rook toolbox
|
|
kubectl --kubeconfig ${KUBE_CONFIG} exec \
|
|
-n ${ROOK_CEPH_NAMESPACE} deploy/${ROOK_CEPH_TOOLS_NAME} -- \
|
|
bash -c "cd ${CMD_DIR} && /usr/bin/ceph ${ARGS}"
|
|
RETURN_CODE=$?
|
|
|
|
if [ ${RETURN_CODE} -ne 0 ]; then
|
|
exit ${RETURN_CODE}
|
|
fi
|
|
|
|
if [[ $OUTPUT_ARG ]]; then
|
|
kubectl --kubeconfig ${KUBE_CONFIG} cp -n ${ROOK_CEPH_NAMESPACE} \
|
|
"${ROOK_CEPH_TOOLS_POD}":"${OUTPUT_REMOTE:1}" "${OUTPUT_ARG}"
|
|
RETURN_CODE=$?
|
|
fi
|
|
exit ${RETURN_CODE}
|
|
fi
|
|
elif [ -f "${NODE_CEPH_CONFIGURED_FLAG}" ]; then
|
|
if [ "${COMPLETION}" = true ]; then
|
|
/usr/bin/ceph "${ARGS[@]}" ''
|
|
else
|
|
/usr/bin/ceph "${ARGS[@]}"
|
|
fi
|
|
else
|
|
LOG "Ceph not enabled."
|
|
fi
|