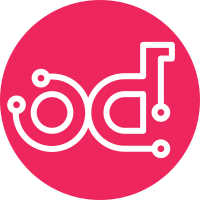
This review enables the E1101 no-member pylint error check and provides fixes for all the places that were broken. There have already been several bugs raised and fixed for code that would have been caught if this error check had been enabled. Filtered files: - The sqlalchemy 'Table' class confuses pylint and has been added to the ignored-classes list along with any sqlalchemy Enums declared in the migration scripts. Removed: - testpci was an old test script and has been removed. - sysinv_deploy_helper was an old helper script that was unused and would have raised runtime errors if invoked. - test_sysinv_deploy_helper.py mocked everything in sysinv_deploy_helper so was removed with it. Fixed: - tox should have been pointing at python-cephclient in stx-integ rather than a completely different codebase on pypi. - impl_zmq / zmq_receiver / matchmaker_redis were removed since only impl_kombu is used by sysinv, and those files are unused pre-oslo code. - configure_osd_pools was never being called and had been partially removed. Now the methods calling the non-existent method are removed as well. (conductor/ceph, conductor/manager, conductor/rpcapi - v1/base.py is an abstract class that requires all of its subclasses to initialize a 'fields' attribute. - v1/certificate v1/storage_ceph_external.py and v1/license were referencing a non-existent 'value' attribute when trying to get a string representation of the exception. - v1/collection has an accessor for _type which is a required field on all subclasses. - v1/lldp_tlv had an invalid check. The class is never instantiated with a from_ihosts field, so that check would always raise an exception. - v1/service_parameter was raising an exception that did not exist. - v1/storage.py was not handling a patch with a for_tierid - v1/user.py; exception.KeyError does not exist. (it is exceptions.KeyError) however exceptions is a default imported module so does not need to be explicitly declared. - v1/utils.py needed to accomodate the renaming of swift to radosgw constants as part of changeid: Id8d5c6b1159881d44810fc3622990456f1e54e75 - objects/* Just about every DB object declaration defines its fields using a dynamic constructor in the base class, so any use of one of those fields to access the id/uuid during the save routine needed to have this no-member check suppressed. - helm/base LABEL_COMPUTE does not exist. It needs to be LABEL_COMPUTE_LABEL - helm/openstack and helm/elastic require all of their subclasses to declare a CHART property however did not enforce themselves as abstract. - puppet/ceph was calling an internal method with the wrong spelling. it is unlikely those methods are being called and can likely be removed. - puppet/ovs was referencing a stale constant. The method that had this typo does not look to be used and can likely be removed. - conductor/ceph: constructing a wrong exception - conductor/manager: Fixed spelling of method: report_external_config_failure. Removed a call to a non-existent ceph method for a non-existent ceph service param. - conductor/openstack: fixed typo in name of get keystone client method - common/exceptions There were exceptions being created that had never been declared in this file so they have been added. This includes: AddressPoolNotFoundByID, NTPNotFound - common/periodictask had a metaclass defined in an unusual way that the subclass was unable to tell that it inherited some attributes - common/policy uses a metaclass to define a variable that confuses pylint - common/setup needed to reference the right module for email MessageError - service_parameter - _rpm_pkg_is_installed was no longer needed since the checking for lefthandclient and 3parclient code was removed from sysinv. - sqlalchemy/api had several typos for classes defined in the db model. There were places that would check isinstance against an internal tablename value, rather than the python class. - sqlalchemy/session uses a attribute system that confuses pylint. Change-Id: If075893067b28b4fb4252ffd12ecef018e890b95 Signed-off-by: Al Bailey <Al.Bailey@windriver.com>
35 lines
920 B
Python
35 lines
920 B
Python
#
|
|
# Copyright (c) 2013-2016 Wind River Systems, Inc.
|
|
#
|
|
# SPDX-License-Identifier: Apache-2.0
|
|
#
|
|
|
|
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
# coding=utf-8
|
|
#
|
|
|
|
from sysinv.objects import base
|
|
from sysinv.objects import utils
|
|
from sysinv.objects import port
|
|
|
|
|
|
class EthernetPort(port.Port):
|
|
|
|
fields = dict({
|
|
'mac': utils.str_or_none,
|
|
'mtu': utils.int_or_none,
|
|
'speed': utils.int_or_none,
|
|
'link_mode': utils.str_or_none,
|
|
'duplex': utils.int_or_none,
|
|
'autoneg': utils.str_or_none,
|
|
'bootp': utils.str_or_none},
|
|
**port.Port.fields)
|
|
|
|
@base.remotable_classmethod
|
|
def get_by_uuid(cls, context, uuid):
|
|
return cls.dbapi.ethernet_port_get(uuid)
|
|
|
|
def save_changes(self, context, updates):
|
|
self.dbapi.ethernet_port_update(self.uuid, # pylint: disable=no-member
|
|
updates)
|