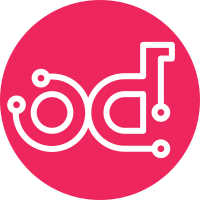
Add an error message to registry-image-delete, currently when users attempt to delete a non-existent image, the command fails silently. This review is adding an error message to this operation, now when the user tries to delete an image that doesn't exist it will be displayed this message: "No tag found for image: <image_name>" Also improving 'system registry-image-list' by adding an optional argument "--filter-out-untagged" to filter and display only images that have associated tags in the system registry. Test Plan: PASS: Run 'system registry-image-list' without '--filter-out-untagged'. PASS: Run 'system registry-image-list' with '--filter-out-untagged' and verify if the untagged images are out of the list. PASS: Delete an image that doesn't exist and verify if it will return the error message. PASS: Delete an existing image and verify if it was successfully deleted. Closes-Bug:2037552 Signed-off-by: Karla Felix <karla.karolinenogueirafelix@windriver.com> Change-Id: Ibbc25dff5f4c1b22d6bf923235e6d0cd821b4953
50 lines
1.5 KiB
Python
50 lines
1.5 KiB
Python
#
|
|
# Copyright (c) 2019 Wind River Systems, Inc.
|
|
#
|
|
# SPDX-License-Identifier: Apache-2.0
|
|
#
|
|
# -*- encoding: utf-8 -*-
|
|
#
|
|
|
|
from cgtsclient.common import base
|
|
from cgtsclient.v1 import options
|
|
|
|
|
|
class RegistryImage(base.Resource):
|
|
def __repr__(self):
|
|
return "<registry_image %s>" % self._info
|
|
|
|
|
|
class RegistryImageManager(base.Manager):
|
|
resource_class = RegistryImage
|
|
|
|
@staticmethod
|
|
def _path(name=None):
|
|
return '/v1/registry_image/%s' % name if name else '/v1/registry_image'
|
|
|
|
def list(self, filter_out_untagged):
|
|
"""Retrieve the list of images from the registry."""
|
|
path = options.build_url(self._path(), None,
|
|
['filter_out_untagged=%s' % filter_out_untagged])
|
|
return self._list(path, 'registry_images')
|
|
|
|
def tags(self, image_name):
|
|
"""Retrieve the list of tags from the registry for a specified image.
|
|
|
|
:param image_name: image name
|
|
"""
|
|
path = options.build_url(self._path(), None, ['image_name=%s' % image_name])
|
|
return self._list(path, 'registry_images')
|
|
|
|
def delete(self, image_name_and_tag):
|
|
"""Delete registry image given name and tag
|
|
|
|
:param image_name_and_tag: a string of the form name:tag
|
|
"""
|
|
path = options.build_url(self._path(), None, ['image_name_and_tag=%s' % image_name_and_tag])
|
|
return self._delete(path)
|
|
|
|
def garbage_collect(self):
|
|
path = options.build_url(self._path(), None, ['garbage_collect=%s' % True])
|
|
return self._create(path, {})
|