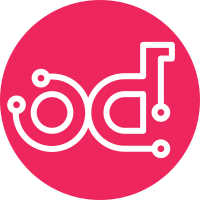
This commit implements access control for DC API. The reference doc can be found at "https://docs.starlingx.io/api-ref/distcloud/api-ref-dcmanager-v1.html". Unit tests and YAML file support will be done in other tasks. The access control implementation for GET requests requires the user to have "reader" role and to be present in either "admin" or "services" project. For other requests, it requires the user to have "admin" role and to be present in either "admin" or "services" project. Requests using public API URLs require no credentials. As all default system users of StarlingX have "admin" role and are present in either project "admin" or "services", there should be no regression with the change introduced here. The implementation done here is a little bit different from the one done for sysinv and FM APIs, because the routing of requests is not done when "before()" method of Pecan hooks are called, so the controller is not defined at this point. To test the access control of DC API, the following commands are used (long list of parameters is replaced by "<params>"): dcmanager subcloud add <params> dcmanager subcloud manage subcloud2 dcmanager subcloud list dcmanager subcloud delete subcloud2 dcmanager subcloud-deploy upload <params> dcmanager subcloud-deploy show dcmanager alarm summary dcmanager patch-strategy create dcmanager patch-strategy show dcmanager patch-strategy apply dcmanager patch-strategy abort dcmanager patch-strategy delete dcmanager strategy-config update <params> subcloud1 dcmanager strategy-config list dcmanager strategy-config delete subcloud1 dcmanager subcloud-group add --name group01 dcmanager subcloud-group update --description test group01 dcmanager subcloud-group list dcmanager subcloud-group delete group01 dcmanager subcloud-backup create --subcloud subcloud1 On test plan, these commands are reffered as "test commands". The access control is not implemented for "dcdbsync" and "dcorch" servers. Also, it is also not implemented for action POST "/v1.0/notifications" in dcmanager API server, as it it is only called indirectly by sysinv controllers. Test Plan: PASS: Successfully deploy a Distributed Cloud (with 1 subcloud) using a CentOS image with this commit present. Successfully create, through openstack CLI, the users: 'testreader' with role 'reader' in project 'admin', 'adminsvc' with role 'admin' in project 'services' and 'otheradmin' with role 'admin' in project 'notadminproject'. Create openrc files for all new users. Note: the other user used is the already existing 'admin' with role 'admin' in project 'admin'. PASS: In the deployed DC, check the behavior of test commands through different users: for "admin" and "adminsvc" users, all commands are successful; for "testreader" user, only the test commands ending with "list" or "summary" (GET requests) are successful; for "otheradmin" user, all commands fail. PASS: In the deployed DC, to assert that public API works without authentication, execute the command "curl -v http://<MGMT_IP>:8119/" and verify that it is accepted and that the HTTP response is 200, and execute the command "curl -v http://<MGMT_IP>:8119/v1.0/subclouds" and verify that it is rejected and that the HTTP response is 401. PASS: In the deployed DC, check through Horizon interface that DC management works correctly with default admin user. Story: 2010149 Task: 46287 Signed-off-by: Joao Victor Portal <Joao.VictorPortal@windriver.com> Change-Id: Icfe24fd62096c7bf0bbb1f97e819dee5aac675e4
30 lines
597 B
Python
30 lines
597 B
Python
#
|
|
# Copyright (c) 2022 Wind River Systems, Inc.
|
|
#
|
|
# SPDX-License-Identifier: Apache-2.0
|
|
#
|
|
|
|
from dcmanager.api.policies import base
|
|
from oslo_policy import policy
|
|
|
|
POLICY_ROOT = 'dc_api:alarm_manager:%s'
|
|
|
|
|
|
alarm_manager_rules = [
|
|
policy.DocumentedRuleDefault(
|
|
name=POLICY_ROOT % 'get',
|
|
check_str='rule:' + base.READER_IN_SYSTEM_PROJECTS,
|
|
description="Get alarms from subclouds.",
|
|
operations=[
|
|
{
|
|
'method': 'GET',
|
|
'path': '/v1.0/alarms'
|
|
}
|
|
]
|
|
)
|
|
]
|
|
|
|
|
|
def list_rules():
|
|
return alarm_manager_rules
|