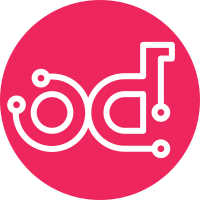
Add support for backing up a subcloud or a group of subclouds using the dcmanager API. The commit includes changes to API and backend dcmanager to validate the user input, create inventory and overrides file and invoke the wrapper playbook for one or multiple subclouds. The wrapper playbook, delivered in a separate commit to playbooks repo [1], will invoke the subcloud backup playbook and transfer backup file as needed to the system controller. In addition, two additional attributes are added to subcloud persistent data: backup_status and backup_datetime. They are included in the dcmanager subcloud related command response [2]. Unit tests will be added in a separate commit. Max parallel operations will be defined during scale testing. Test Plan: PASS: - Ensure tox tests are passing - Issue backup request for a single subcloud - Issue backup request for a small group of subclouds - Check API reachability using manual HTTP request - Check validations on API arguments - Attempt backup on an offline subcloud - Attempt backup on an unmanaged subcloud - Attempt group backup with both valid and invalid subclouds - Verify generated subcloud inventory file - Verify generated overrides file for different option values - Verify generated command for wrapper playbook invoke - Verify wrapper playbook is invoked correctly using a stub - Verify migration script for new backup fields - Check backup_status for failed validation - Check backup_status for failed backup prep - Check backup_status for failed playbook execution - Check backup_status and backup_datetime for successful execution - Test different thread pool sizes for group operation - Attempt backup on subcloud with invalid deploy state - Integration testing with dcmanager-client - Integration testing with wrapper playbook [1] Review for backup wrapper playbook https://review.opendev.org/c/starlingx/ansible-playbooks/+/855082 [2] Review for subcloud backup CLI https://review.opendev.org/c/starlingx/distcloud-client/+/853834 Story: 2010116 Task: 45696 Signed-off-by: Gabriel Silva Trevisan <gabriel.silvatrevisan@windriver.com> Change-Id: Ie9dc02b53583d28e593ce207d3a8da100cbd7bef
69 lines
2.6 KiB
Python
69 lines
2.6 KiB
Python
# Copyright (c) 2017 Ericsson AB.
|
|
# Copyright (c) 2017-2021 Wind River Systems, Inc.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
#
|
|
import pecan
|
|
|
|
from dcmanager.api.controllers.v1 import alarm_manager
|
|
from dcmanager.api.controllers.v1 import notifications
|
|
from dcmanager.api.controllers.v1 import subcloud_backup
|
|
from dcmanager.api.controllers.v1 import subcloud_deploy
|
|
from dcmanager.api.controllers.v1 import subcloud_group
|
|
from dcmanager.api.controllers.v1 import subclouds
|
|
from dcmanager.api.controllers.v1 import sw_update_options
|
|
from dcmanager.api.controllers.v1 import sw_update_strategy
|
|
|
|
|
|
class Controller(object):
|
|
|
|
def _get_resource_controller(self, remainder):
|
|
|
|
if not remainder:
|
|
pecan.abort(404)
|
|
return
|
|
minor_version = remainder[-1]
|
|
remainder = remainder[:-1]
|
|
sub_controllers = dict()
|
|
if minor_version == '0':
|
|
sub_controllers["subclouds"] = subclouds.SubcloudsController
|
|
sub_controllers["subcloud-deploy"] = subcloud_deploy.\
|
|
SubcloudDeployController
|
|
sub_controllers["alarms"] = alarm_manager.SubcloudAlarmController
|
|
sub_controllers["sw-update-strategy"] = \
|
|
sw_update_strategy.SwUpdateStrategyController
|
|
sub_controllers["sw-update-options"] = \
|
|
sw_update_options.SwUpdateOptionsController
|
|
sub_controllers["subcloud-groups"] = \
|
|
subcloud_group.SubcloudGroupsController
|
|
sub_controllers["notifications"] = \
|
|
notifications.NotificationsController
|
|
sub_controllers["subcloud-backup"] = subcloud_backup.\
|
|
SubcloudBackupController
|
|
|
|
for name, ctrl in sub_controllers.items():
|
|
setattr(self, name, ctrl)
|
|
|
|
resource = remainder[0]
|
|
if resource not in sub_controllers:
|
|
pecan.abort(404)
|
|
return
|
|
|
|
remainder = remainder[1:]
|
|
return sub_controllers[resource](), remainder
|
|
|
|
@pecan.expose()
|
|
def _lookup(self, *remainder):
|
|
return self._get_resource_controller(remainder)
|