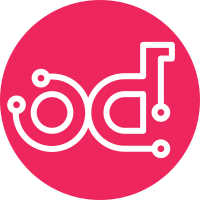
Here is added a hook to the WSGI compliant Pecan server that receives the DC Manager API requests. This hook logs the needed request data to "/var/log/dcmanager/dcmanager-api.log". All requests are logged except the ones of "GET" type. The code is a port from the same hook that exists in "starlingx/config" repository, also called "AuditLogging". Note: there are no "PUT" requests available in DC Manager API v1. Test Plan: PASS: Successfully deploy a Distributed Cloud and verify that the logs of "dcmanager-api" service are present in file "/var/log/dcmanager/dcmanager-api.log". PASS: In the deployed Distributed Cloud, execute command "dcmanager subcloud list" and check that no GET request was logged in "dcmanager-api.log". PASS: In the deployed Distributed Cloud, execute command "dcmanager subcloud-group add --name subcloudgroup1" and check that a "POST /v1.0/subcloud-groups/" request was logged in "dcmanager-api.log" with status "200". PASS: In the deployed Distributed Cloud, execute command "dcmanager subcloud delete subcloud2" and check that a "DELETE /v1.0/subclouds/subcloud2" request was logged in "dcmanager-api.log" with status "404". PASS: In the deployed Distributed Cloud, execute command "dcmanager subcloud update subcloud1 --description 'newdesc'" and check that a "PATCH /v1.0/subclouds/subcloud1" request was logged in "dcmanager-api.log" with status "200". PASS: In the deployed Distributed Cloud, execute command "dcmanager patch-strategy create" and check that a "POST /v1.0/sw-update-strategy/" request was logged in "dcmanager-api.log" with the correct request fields. PASS: In the deployed Distributed Cloud, execute command "dcmanager patch-strategy apply" and check that a "POST /v1.0/sw-update-strategy/actions" request was logged in "dcmanager-api.log" with the correct request fields. PASS: In the deployed Distributed Cloud, execute command "dcmanager patch-strategy delete" and check that a "DELETE /v1.0/sw-update-strategy" request was logged in "dcmanager-api.log" with the correct request fields. PASS: In the deployed Distributed Cloud, execute command "dcmanager subcloud-deploy show" and check that no GET request was logged in "dcmanager-api.log". PASS: In the deployed Distributed Cloud, execute command "dcmanager subcloud-deploy upload" and check that a "POST /v1.0/subcloud-deploy/" request was logged in "dcmanager-api.log" with the correct request fields. PASS: Successfully build package "distributedcloud" of this repository as Debian package. PASS: In the deployed Distributed Cloud, execute command "collect --all" in the central cloud and check that all files inside "/var/log/dcmanager/" are included in the generated "tar" file. Story: 2009824 Task: 44550 Signed-off-by: Joao Victor Portal <Joao.VictorPortal@windriver.com> Change-Id: I3ddbdf486257ad6f64f827746ebc59d570691e3d
96 lines
2.6 KiB
Python
96 lines
2.6 KiB
Python
# Copyright (c) 2015 Huawei, Tech. Co,. Ltd.
|
|
# Copyright (c) 2017, 2019, 2021 Wind River Systems, Inc.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
#
|
|
|
|
import pecan
|
|
|
|
from keystonemiddleware import auth_token
|
|
from oslo_config import cfg
|
|
from oslo_middleware import request_id
|
|
from oslo_service import service
|
|
|
|
from dcmanager.common import context as ctx
|
|
from dcmanager.common.i18n import _
|
|
|
|
|
|
def setup_app(*args, **kwargs):
|
|
|
|
opts = cfg.CONF.pecan
|
|
config = {
|
|
'server': {
|
|
'port': cfg.CONF.bind_port,
|
|
'host': cfg.CONF.bind_host
|
|
},
|
|
'app': {
|
|
'root': 'dcmanager.api.controllers.root.RootController',
|
|
'modules': ['dcmanager.api'],
|
|
"debug": opts.debug,
|
|
"auth_enable": opts.auth_enable,
|
|
'errors': {
|
|
400: '/error',
|
|
'__force_dict__': True
|
|
}
|
|
}
|
|
}
|
|
|
|
pecan_config = pecan.configuration.conf_from_dict(config)
|
|
|
|
# app_hooks = [], hook collection will be put here later
|
|
|
|
app = pecan.make_app(
|
|
pecan_config.app.root,
|
|
debug=False,
|
|
wrap_app=_wrap_app,
|
|
force_canonical=False,
|
|
hooks=lambda: [
|
|
ctx.AuthHook(),
|
|
ctx.AuditLoggingHook()
|
|
],
|
|
guess_content_type_from_ext=True
|
|
)
|
|
|
|
return app
|
|
|
|
|
|
def _wrap_app(app):
|
|
app = request_id.RequestId(app)
|
|
if cfg.CONF.pecan.auth_enable and cfg.CONF.auth_strategy == 'keystone':
|
|
conf = dict(cfg.CONF.keystone_authtoken)
|
|
# Change auth decisions of requests to the app itself.
|
|
conf.update({'delay_auth_decision': True})
|
|
|
|
# NOTE: Policy enforcement works only if Keystone
|
|
# authentication is enabled. No support for other authentication
|
|
# types at this point.
|
|
return auth_token.AuthProtocol(app, conf)
|
|
else:
|
|
return app
|
|
|
|
|
|
_launcher = None
|
|
|
|
|
|
def serve(api_service, conf, workers=1):
|
|
global _launcher
|
|
if _launcher:
|
|
raise RuntimeError(_('serve() can only be called once'))
|
|
|
|
_launcher = service.launch(conf, api_service, workers=workers)
|
|
|
|
|
|
def wait():
|
|
_launcher.wait()
|