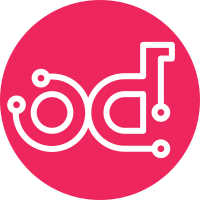
This commit enables the check of new pylint/pep8 violations. PYLINT - All convention related checks, except: - missing-class-docstring - missing-function-docstring - missing-module-docstring - consider-using-f-string - invalid-name - import-outside-toplevel - too-many-lines - consider-iterating-dictionary - unnecessary-lambda-assignment PEP8: - E117: over-indented - E123: closing bracket does not match indentation of opening bracket's line - E125: continuation line with the same indent as the next logical line - E305: expected 2 blank lines after class or function definition - E402: module level import not at top of file - E501: line too long - H216: flag use of third party mock Test Plan: 1. Verify that all Tox tests pass without errors: - tox -e py39,pylint,pep8 Partial-bug: 2033294 Change-Id: I834d15ae1df6b2b449502dcb0bcf0c359a99514f Signed-off-by: Hugo Brito <hugo.brito@windriver.com>
98 lines
3.4 KiB
Python
98 lines
3.4 KiB
Python
# Copyright (c) 2015 Ericsson AB.
|
|
# Copyright (c) 2024 Wind River Systems, Inc.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""Resource object."""
|
|
|
|
from oslo_versionedobjects import base as ovo_base
|
|
from oslo_versionedobjects import fields as ovo_fields
|
|
|
|
from dcorch.common import exceptions
|
|
from dcorch.db import api as db_api
|
|
from dcorch.objects import base
|
|
|
|
|
|
@base.OrchestratorObjectRegistry.register
|
|
class Resource(base.OrchestratorObject, base.VersionedObjectDictCompat):
|
|
"""DC Orchestrator subcloud object."""
|
|
|
|
fields = {
|
|
'id': ovo_fields.IntegerField(),
|
|
'uuid': ovo_fields.UUIDField(),
|
|
'resource_type': ovo_fields.StringField(),
|
|
'master_id': ovo_fields.StringField(),
|
|
}
|
|
|
|
def create(self):
|
|
if self.obj_attr_is_set('id'):
|
|
raise exceptions.ObjectActionError(action='create',
|
|
reason='already created')
|
|
updates = self.obj_get_changes()
|
|
try:
|
|
resource_type = updates.pop('resource_type')
|
|
except KeyError:
|
|
raise exceptions.ObjectActionError(
|
|
action="create",
|
|
reason="cannot create a Resource object without a "
|
|
"resource_type set")
|
|
|
|
db_resource = db_api.resource_create(
|
|
self._context, resource_type, updates)
|
|
return self._from_db_object(self._context, self, db_resource)
|
|
|
|
@classmethod
|
|
def get_by_type_and_master_id(cls, context, resource_type, master_id):
|
|
db_resource = db_api.resource_get_by_type_and_master_id(
|
|
context, resource_type, master_id)
|
|
return cls._from_db_object(context, cls(), db_resource)
|
|
|
|
@classmethod
|
|
def get_by_id(cls, context, id):
|
|
db_resource = db_api.resource_get_by_id(context, id)
|
|
return cls._from_db_object(context, cls(), db_resource)
|
|
|
|
def delete(self):
|
|
db_api.resource_delete(
|
|
self._context,
|
|
self.resource_type, # pylint: disable=E1101
|
|
self.master_id) # pylint: disable=E1101
|
|
|
|
def save(self):
|
|
updates = self.obj_get_changes()
|
|
updates.pop('id', None)
|
|
updates.pop('uuid', None)
|
|
db_resource = db_api.resource_update(self._context,
|
|
self.id, # pylint: disable=E1101
|
|
updates)
|
|
self._from_db_object(self._context, self, db_resource)
|
|
self.obj_reset_changes()
|
|
|
|
|
|
@base.OrchestratorObjectRegistry.register
|
|
class ResourceList(ovo_base.ObjectListBase, base.OrchestratorObject):
|
|
"""DC Orchestrator resource list object."""
|
|
VERSION = '1.1'
|
|
|
|
fields = {
|
|
'objects': ovo_fields.ListOfObjectsField('Resource'),
|
|
}
|
|
|
|
@classmethod
|
|
def get_all(cls, context, resource_type=None):
|
|
resources = db_api.resource_get_all(
|
|
context, resource_type)
|
|
return ovo_base.obj_make_list(
|
|
context, cls(context), Resource, resources)
|