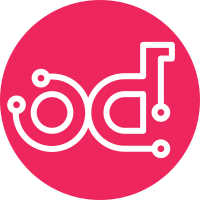
This part one of a two part HA Improvements feature that introduces the collection of heartbeat health at the system level. The full feature is intended to provide service management (SM) with the last 2 seconds of maintenace's heartbeat health view that is reflective of each controller's connectivity to each host including its peer controller. The heartbeat cluster summary information is additional information for SM to draw on when needing to make a choice of which controller is healthier, if/when to switch over and to ultimately avoid split brain scenarios in a two controller system. Feature Behavior: A common heartbeat cluster data structure is introduced and published to the sysroot for SM. The heartbeat service populates and maintains a local copy of this structure with data that reflects the responsivness for each monitored network of all the monitored hosts for the last 20 heartbeat periods. Mtce sends the current cluster summary to SM upon request. General flow of cluster feature wrt hbsAgent: hbs_cluster_init: general data init hbs_cluster_nums: set controller and network numbers forever: select: hbs_cluster_add / hbs_cluster_del: - add/del hosts from mtcAgent hbs_sm_handler -> hbs_cluster_send: - send cluster to SM heartbeating: hbs_cluster_append: add controller cluster to pulse request hbs_cluster_update: get controller cluster data from pulse responses hbs_cluster_save: save other controller cluster view in cluster vault hbs_cluster_log: log cluster state changes (clog) Test Plan: PASS: Verify compute system install PASS: Verify storage system install PASS: Verify cluster data ; all members of structure PASS: Verify storage-0 state management PASS: Verify add of second controller PASS: Verify add of storage-0 node PASS: Verify behavior over Swact PASS: Verify lock/unlock of second controller ; overall behavior PASS: Verify lock/unlock of storage-0 ; overall behavior PASS: Verify lock/unlock of storage-1 ; overall behavior PASS: Verify lock/unlock of compute nodes ; overall behavior PASS: Verify heartbeat failure and recovery of compute node PASS: Verify heartbeat failure and recovery of storage-0 PASS: Verify heartbeat failure and recovery of controller PASS: Verify delete of controller node PASS: Verify delete of storage-0 PASS: Verify delete of compute node PASS: Verify cluster when controller-1 active / controller-0 disabled PASS: Verify MNFA and recovery handling PASS: Verify handling in presence of multiple failure conditions PASS: Verify hbsAgent memory leak soak test with continuous SM query. PASS: Verify active controller-1 infra network failure behavior. PASS: Verify inactive controller-1 infra network failure behavior. Change-Id: I4154287f6dcf5249be5ab3180f2752ab47c5da3c Story: 2003576 Task: 24907 Signed-off-by: Eric MacDonald <eric.macdonald@windriver.com>
165 lines
6.6 KiB
C++
165 lines
6.6 KiB
C++
#ifndef __INCLUDE_JSONUTIL_H__
|
|
#define __INCLUDE_JSONUTIL_H__
|
|
/*
|
|
* Copyright (c) 2013, 2016 Wind River Systems, Inc.
|
|
*
|
|
* SPDX-License-Identifier: Apache-2.0
|
|
*
|
|
*/
|
|
|
|
/**
|
|
* @file
|
|
* Wind River CGTS Platform Controller Maintenance
|
|
*
|
|
* JSON Utility Header
|
|
*/
|
|
|
|
#include <iostream>
|
|
#include <list>
|
|
#include "json-c/json.h"
|
|
|
|
using namespace std;
|
|
|
|
/** Inventory json GET request load struct.
|
|
*
|
|
* Used to hold/load the parsed contents of the response
|
|
* json string returned from an inventory HTTP GET request. */
|
|
typedef struct
|
|
{
|
|
int elements ; /**< converted elements */
|
|
/** An array of inventory information, one for each host */
|
|
node_inv_type host[MAX_JSON_INV_GET_HOST_NUM];
|
|
string next ; /**< pointer to the next inventory element */
|
|
} jsonUtil_info_type ;
|
|
|
|
#define MTC_JSON_AUTH_TOKEN "token"
|
|
#define MTC_JSON_AUTH_SVCCAT "catalog"
|
|
#define MTC_JSON_AUTH_TYPE "type" /**< looking for "compute" */
|
|
#define MTC_JSON_AUTH_ENDPOINTS "endpoints"
|
|
#define MTC_JSON_AUTH_URL "url"
|
|
#define MTC_JSON_AUTH_ISSUE "issued_at"
|
|
#define MTC_JSON_AUTH_EXPIRE "expires_at"
|
|
#define MTC_JSON_AUTH_ID "X-Subject-Token"
|
|
#define MTC_JSON_AUTH_COMP "compute"
|
|
#define MTC_JSON_AUTH_INTERFACE "interface" /** looking for admin */
|
|
#define MTC_JSON_AUTH_ADMIN "admin"
|
|
|
|
|
|
/** Authroization info loaded from the authorization server */
|
|
typedef struct
|
|
{
|
|
bool updated ; /**< true if struct has been updated. */
|
|
int status ; /**< PASS or error code. token is only valid if PASS.*/
|
|
string tokenid ; /**< The long encrypted toke.n */
|
|
string issued ; /**< The "issued_at": "<date-time>". */
|
|
string expiry ; /**< The "expires": "<date-time>". */
|
|
string adminURL; /**< path to the nova server. */
|
|
} jsonUtil_auth_type ;
|
|
|
|
/** Module initialization interface.
|
|
*/
|
|
void jsonUtil_init ( jsonUtil_info_type & info );
|
|
|
|
/** Print the authroization struct to stdio.
|
|
*/
|
|
void jsonUtil_print ( jsonUtil_info_type & info , int index );
|
|
void jsonUtil_print_inv ( node_inv_type & info );
|
|
|
|
int jsonUtil_get_key_val ( char * json_str_ptr,
|
|
string key,
|
|
string & value );
|
|
|
|
int jsonUtil_get_key_val_int ( char * json_str_ptr,
|
|
string key,
|
|
int & value );
|
|
|
|
/** Submit a request to get an authorization token and nova URL */
|
|
int jsonApi_auth_request ( string & hostname, string & payload );
|
|
|
|
/** Parse through the authorization request's response json string
|
|
* and load the relavent information into the passed in structure */
|
|
int jsonUtil_inv_load ( char * json_str_ptr,
|
|
jsonUtil_info_type & info );
|
|
|
|
int jsonUtil_load_host ( char * json_str_ptr, node_inv_type & info );
|
|
int jsonUtil_load_host_state ( char * json_str_ptr, node_inv_type & info );
|
|
|
|
int jsonUtil_hwmon_info ( char * json_str_ptr, node_inv_type & info );
|
|
|
|
/** Handle the patch request response and verify execution status */
|
|
int jsonUtil_patch_load ( char * json_str_ptr, node_inv_type & info );
|
|
|
|
/** Tokenizes the json string and loads 'info' with the received token
|
|
*
|
|
* @param json_str_ptr
|
|
* to a json string
|
|
* @param info
|
|
* is the updated jsonUtil_auth_type bucket containing the token
|
|
*
|
|
* @return execution status (PASS or FAIL)
|
|
*
|
|
*- PASS indicates tokenization ok and info is updated.
|
|
*- FAIL indicates bad or error reply in json string.
|
|
*
|
|
*/
|
|
int jsonApi_auth_load ( string & hostname, char * json_str_ptr,
|
|
jsonUtil_auth_type & info );
|
|
|
|
|
|
/***************************************************************************
|
|
* This utility searches for an 'array_label' and then loops over the array
|
|
* looking at each element for the specified 'search_key' and 'search_value'
|
|
* Once found it searches that same element for the specified 'element_key'
|
|
* and loads its value content into 'element_value' - what we're looking for
|
|
***************************************************************************/
|
|
int jsonApi_array_value ( char * json_str_ptr,
|
|
string array_label,
|
|
string search_key,
|
|
string search_value,
|
|
string element_key,
|
|
string & element_value);
|
|
|
|
/***********************************************************************
|
|
* This utility updates the reference key_list with all the
|
|
* values for the specified label.
|
|
***********************************************************************/
|
|
int jsonUtil_get_list ( char * json_str_ptr,
|
|
string label, list<string> & key_list );
|
|
|
|
/***********************************************************************
|
|
* This utility updates the reference element with the number of array
|
|
* elements for the specified label in the provided string
|
|
***********************************************************************/
|
|
int jsonUtil_array_elements ( char * json_str_ptr, string label, int & elements );
|
|
|
|
/***********************************************************************
|
|
* This utility updates the reference string 'element' with the
|
|
* contents of the specified labeled array element index.
|
|
***********************************************************************/
|
|
int jsonUtil_get_array_idx ( char * json_str_ptr, string label, int idx, string & element );
|
|
|
|
/***********************************************************************
|
|
* Escape special characters in JSON string
|
|
************************************************************************/
|
|
string jsonUtil_escapeSpecialChar(const string& input);
|
|
|
|
int jsonUtil_get_key_value_int ( struct json_object * obj, const char * key );
|
|
bool jsonUtil_get_key_value_bool ( struct json_object * obj, const char * key );
|
|
string jsonUtil_get_key_value_string ( struct json_object * obj, const char * key );
|
|
|
|
/***********************************************************************
|
|
* Get JSON Integer Value from Key
|
|
* return 0 if success, -1 if fail.
|
|
***********************************************************************/
|
|
int jsonUtil_get_int( struct json_object *jobj,
|
|
const char *key, void *value );
|
|
|
|
/***********************************************************************
|
|
* Get JSON String Value from Key
|
|
* return 0 if success, -1 if fail.
|
|
***********************************************************************/
|
|
int jsonUtil_get_string( struct json_object* jobj,
|
|
const char* key, string * value );
|
|
|
|
#endif /* __INCLUDE_JSONUTIL_H__ */
|