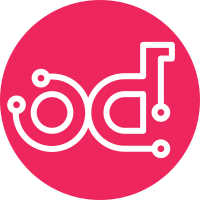
tb.sh create might fail to create the builder docker image. Yum install of the mock package failed, but yum did not report the failure because other packages in the instalation set succeeded. A subsequent command in the dockerfile fails when it tries to remove/relocate /var/lib/mock, but failes because it is not present. The yum error reporting was corrected in a recent update. But this does not address cached copies of old and broken yum install steps that pre-date the fix. The mock package is paricularly sensitive as it has cengn as the only source, where as other packages have multiple sources. One option is to force docker to not use the cache at all, which is slow. The second option is to change the docker file, placing the yum command to install mock under a seperate docker RUN command. The altered build instructions ensure that the docker cache with the broken install can't be used. While we are at it, move the user/project customization steps as far down as possible to improve cache usage. This change implements both. Closes-Bug: 1917901 Signed-off-by: Scott Little <scott.little@windriver.com> Change-Id: I28041bb44af53384c00a750b7162c6c6808c4e2d
142 lines
3.5 KiB
Bash
Executable File
142 lines
3.5 KiB
Bash
Executable File
#!/bin/bash
|
|
# tb.sh - tbuilder commands
|
|
#
|
|
# Subcommands:
|
|
# env - Display a selection of configuration values
|
|
# exec - Starts a shell inside a running container
|
|
# run - Starts a container
|
|
# stop - Stops a running container
|
|
#
|
|
# Configuration
|
|
# tb.sh expects to find its configuration file buildrc in the current
|
|
# directory, like Vagrant looks for Vagrantfile.
|
|
|
|
SCRIPT_DIR=$(cd $(dirname "$0") && pwd)
|
|
WORK_DIR=$(pwd)
|
|
|
|
# Load tbuilder configuration
|
|
if [[ -r ${WORK_DIR}/buildrc ]]; then
|
|
source ${WORK_DIR}/buildrc
|
|
fi
|
|
|
|
CMD=$1
|
|
|
|
TC_CONTAINER_NAME=${MYUNAME}-centos-builder
|
|
TC_CONTAINER_TAG=local/${MYUNAME}-stx-builder:7.8
|
|
TC_DOCKERFILE=Dockerfile
|
|
NO_CACHE=0
|
|
|
|
function create_container {
|
|
local EXTRA_ARGS=""
|
|
|
|
if [ ! -z ${MY_EMAIL} ]; then
|
|
EXTRA_ARGS+="--build-arg MY_EMAIL=${MY_EMAIL}"
|
|
fi
|
|
|
|
if [ $NO_CACHE -eq 1 ]; then
|
|
EXTRA_ARGS+=" --no-cache"
|
|
fi
|
|
|
|
docker build \
|
|
--build-arg MYUID=$(id -u) \
|
|
--build-arg MYUNAME=${USER} \
|
|
${EXTRA_ARGS} \
|
|
--ulimit core=0 \
|
|
--network host \
|
|
-t ${TC_CONTAINER_TAG} \
|
|
-f ${TC_DOCKERFILE} \
|
|
.
|
|
}
|
|
|
|
function exec_container {
|
|
echo "docker cp ${WORK_DIR}/buildrc ${TC_CONTAINER_NAME}:/home/${MYUNAME}"
|
|
docker cp ${WORK_DIR}/buildrc ${TC_CONTAINER_NAME}:/home/${MYUNAME}
|
|
docker cp ${WORK_DIR}/localrc ${TC_CONTAINER_NAME}:/home/${MYUNAME}
|
|
docker exec -it --user=${MYUNAME} -e MYUNAME=${MYUNAME} ${TC_CONTAINER_NAME} script -q -c "/bin/bash" /dev/null
|
|
}
|
|
|
|
function run_container {
|
|
# create localdisk
|
|
mkdir -p ${LOCALDISK}/designer/${MYUNAME}/${PROJECT}
|
|
#create centOS mirror
|
|
mkdir -p ${HOST_MIRROR_DIR}/CentOS
|
|
|
|
docker run -it --rm \
|
|
--name ${TC_CONTAINER_NAME} \
|
|
--detach \
|
|
-v /var/run/docker.sock:/var/run/docker.sock \
|
|
-v $(readlink -f ${LOCALDISK}):/${GUEST_LOCALDISK} \
|
|
-v ${HOST_MIRROR_DIR}:/import/mirrors:ro \
|
|
-v /sys/fs/cgroup:/sys/fs/cgroup:ro \
|
|
-v ~/.ssh:/mySSH:ro \
|
|
--tmpfs /tmp \
|
|
--tmpfs /run \
|
|
-e "container=docker" \
|
|
-e MYUNAME=${MYUNAME} \
|
|
--privileged=true \
|
|
--security-opt seccomp=unconfined \
|
|
${TC_CONTAINER_TAG}
|
|
}
|
|
|
|
function stop_container {
|
|
docker stop ${TC_CONTAINER_NAME}
|
|
}
|
|
|
|
function kill_container {
|
|
docker kill ${TC_CONTAINER_NAME}
|
|
}
|
|
|
|
function clean_container {
|
|
docker rm ${TC_CONTAINER_NAME} || true
|
|
docker image rm ${TC_CONTAINER_TAG}
|
|
}
|
|
|
|
function usage {
|
|
echo "$0 [create|create_no_cache|run|exec|env|stop|kill|clean]"
|
|
}
|
|
|
|
case $CMD in
|
|
env)
|
|
echo "LOCALDISK=${LOCALDISK}"
|
|
echo "GUEST_LOCALDISK=${GUEST_LOCALDISK}"
|
|
echo "TC_DOCKERFILE=${TC_DOCKERFILE}"
|
|
echo "TC_CONTAINER_NAME=${TC_CONTAINER_NAME}"
|
|
echo "TC_CONTAINER_TAG=${TC_CONTAINER_TAG}"
|
|
echo "SOURCE_REMOTE_NAME=${SOURCE_REMOTE_NAME}"
|
|
echo "SOURCE_REMOTE_URI=${SOURCE_REMOTE_URI}"
|
|
echo "HOST_MIRROR_DIR=${HOST_MIRROR_DIR}"
|
|
echo "MY_RELEASE=${MY_RELEASE}"
|
|
echo "MY_REPO_ROOT_DIR=${MY_REPO_ROOT_DIR}"
|
|
echo "LAYER=${LAYER}"
|
|
echo "MYUNAME=${MYUNAME}"
|
|
echo "MY_EMAIL=${MY_EMAIL}"
|
|
;;
|
|
create)
|
|
create_container
|
|
;;
|
|
create_no_cache)
|
|
NO_CACHE=1
|
|
create_container
|
|
;;
|
|
exec)
|
|
exec_container
|
|
;;
|
|
run)
|
|
run_container
|
|
;;
|
|
stop)
|
|
stop_container
|
|
;;
|
|
kill)
|
|
kill_container
|
|
;;
|
|
clean)
|
|
clean_container
|
|
;;
|
|
*)
|
|
echo "Unknown command: $CMD"
|
|
usage
|
|
exit 1
|
|
;;
|
|
esac
|