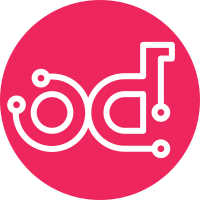
VMwareGuestInfoService is a metadata service which uses VMware's rpctool to extract guest metadata and userdata configured for machines running on VMware hypervisors. The implementation is similar to: https://github.com/vmware/cloud-init-vmware-guestinfo Supported features for the metadata service: * instance id * hostname * admin username * admin password * public SSH keys * userdata Configuration options: ```ini [vmwarequestinfo] vmware_rpctool_path=%ProgramFiles%/VMware/VMware Tools/rpctool.exe ``` The VMware RPC tool used to query the instance metadata and userdata needs to be present at the config option path. Both json and yaml are supported as metadata formats. The metadata / userdata can be encoded in base64, gzip or gzip+base64. Example metadata in yaml format: ```yaml instance-id: cloud-vm local-hostname: cloud-vm admin-username: cloud-username admin-password: Passw0rd public-keys-data: | ssh-key 1 ssh-key 2 ``` This metadata content needs to be sent as string in the guestinfo dictionary, thus needs to be converted to base64 (it is recommended to gzip it too). To convert to gzip+base64 format: ```bash cat metadata.yml | gzip.exe -9 | base64.exe -w0 ``` Co-Authored-By: Rui Lopes <rgl@ruilopes.com> Change-Id: I6a8430e87ee03d2e8fdd2685b05e60c5c0ffb5be
47 lines
1.7 KiB
Python
47 lines
1.7 KiB
Python
# Copyright 2020 Cloudbase Solutions Srl
|
|
# Copyright 2019 ruilopes.com
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""Config options available for the VMware metadata service."""
|
|
|
|
from oslo_config import cfg
|
|
|
|
from cloudbaseinit.conf import base as conf_base
|
|
|
|
|
|
class VMwareGuestInfoConfigOptions(conf_base.Options):
|
|
|
|
"""Config options available for the VMware GuestInfo metadata service."""
|
|
|
|
def __init__(self, config):
|
|
super(VMwareGuestInfoConfigOptions, self).__init__(
|
|
config, group="vmwareguestinfo")
|
|
self._options = [
|
|
cfg.StrOpt(
|
|
'vmware_rpctool_path',
|
|
default="%ProgramFiles%/VMware/VMware Tools/rpctool.exe",
|
|
help='The local path where VMware rpctool is found')
|
|
]
|
|
|
|
def register(self):
|
|
"""Register the current options to the global ConfigOpts object."""
|
|
group = cfg.OptGroup(self.group_name,
|
|
title='VMware GuestInfo Options')
|
|
self._config.register_group(group)
|
|
self._config.register_opts(self._options, group=group)
|
|
|
|
def list(self):
|
|
"""Return a list which contains all the available options."""
|
|
return self._options
|