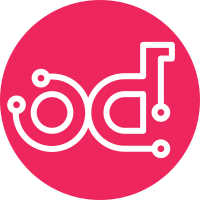
This commit adds the idempotent id decorator and the corresponding tooling from the tempest repo At the time of this migration the current head on each tempest file is: check_uuid.py: I1948c2d038d89af8dcfe69d01dcc49d024ae3210 test.py: Id2bcabb97c61f68dbcee5afbfaf9d27c8f52264c test_decorators.py: I24f6e20cc8e310ba69fb23510795e235218abb2d To get a complete history of the changes from tempest look up those change ids from the tempest repo as a starting point. Change-Id: Id0611ce8c1ccb319313959aa865dd91741295eb2
62 lines
2.1 KiB
Python
62 lines
2.1 KiB
Python
# Copyright 2015 Hewlett-Packard Development Company, L.P.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import functools
|
|
import uuid
|
|
|
|
import six
|
|
import testtools
|
|
|
|
|
|
def skip_because(*args, **kwargs):
|
|
"""A decorator useful to skip tests hitting known bugs
|
|
|
|
@param bug: bug number causing the test to skip
|
|
@param condition: optional condition to be True for the skip to have place
|
|
"""
|
|
def decorator(f):
|
|
@functools.wraps(f)
|
|
def wrapper(self, *func_args, **func_kwargs):
|
|
skip = False
|
|
if "condition" in kwargs:
|
|
if kwargs["condition"] is True:
|
|
skip = True
|
|
else:
|
|
skip = True
|
|
if "bug" in kwargs and skip is True:
|
|
if not kwargs['bug'].isdigit():
|
|
raise ValueError('bug must be a valid bug number')
|
|
msg = "Skipped until Bug: %s is resolved." % kwargs["bug"]
|
|
raise testtools.TestCase.skipException(msg)
|
|
return f(self, *func_args, **func_kwargs)
|
|
return wrapper
|
|
return decorator
|
|
|
|
|
|
def idempotent_id(id):
|
|
"""Stub for metadata decorator"""
|
|
if not isinstance(id, six.string_types):
|
|
raise TypeError('Test idempotent_id must be string not %s'
|
|
'' % type(id).__name__)
|
|
uuid.UUID(id)
|
|
|
|
def decorator(f):
|
|
f = testtools.testcase.attr('id-%s' % id)(f)
|
|
if f.__doc__:
|
|
f.__doc__ = 'Test idempotent id: %s\n%s' % (id, f.__doc__)
|
|
else:
|
|
f.__doc__ = 'Test idempotent id: %s' % id
|
|
return f
|
|
return decorator
|