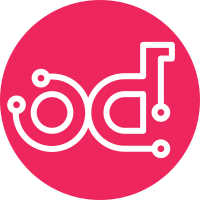
We have plugin for LVM usage in k8s, this patch adds basic test using this plugin. This test creates an environment (which should have extra storage), installs required software to use LVM on nodes, uploads a plugin to each node and then creates nginx pod using this plugin to create its storage. Sample command to run test: ENV_NAME="lvm_usage"\ CONF_PATH=fuel_ccp_tests/templates/default-with-storage.yaml\ IMAGE_PATH=/path/to/image LVM_PLUGIN_PATH=/path/to/plugin/binary\ DEPLOY_SCRIPT=/path/to/fuel-ccp-installer/utils/jenkins/kargo_deploy.sh\ py.test -s fuel_ccp_tests/tests/system/test_k8s_lvm_plugin_usage.py Change-Id: I0a31324e445902774f35b6cf2af1d9d43e74f53e
76 lines
3.0 KiB
Python
76 lines
3.0 KiB
Python
# Copyright 2016 Mirantis, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from fuel_ccp_tests import logger
|
|
|
|
LOG = logger.logger
|
|
LOG.addHandler(logger.console)
|
|
|
|
|
|
class SystemBaseTest(object):
|
|
"""SystemBaseTest contains setup/teardown for environment creation"""
|
|
|
|
def calico_ipip_exists(self, underlay):
|
|
"""Check if ipip is in calico pool config
|
|
|
|
:param underlay: fuel_ccp_tests.managers.UnderlaySSHManager
|
|
"""
|
|
cmd = "calicoctl pool show | grep ipip"
|
|
for node_name in underlay.node_names():
|
|
underlay.sudo_check_call(cmd, node_name=node_name)
|
|
|
|
def required_images_exists(self, node_name, underlay, required_images):
|
|
"""Check if there are all base containers on node
|
|
|
|
:param node_name: string
|
|
:param underlay: fuel_ccp_tests.managers.UnderlaySSHManager
|
|
:param required_images: list
|
|
"""
|
|
cmd = "docker ps --no-trunc --format '{{.Image}}'"
|
|
result = underlay.sudo_check_call(cmd, node_name=node_name)
|
|
images = [x.split(":")[0] for x in result['stdout']]
|
|
assert set(required_images) < set(images),\
|
|
"Running containers check failed on node '{}'".format(node_name)
|
|
|
|
def check_list_required_images(self, underlay, required_images):
|
|
"""Check running containers on each node
|
|
|
|
:param underlay: fuel_ccp_tests.managers.UnderlaySSHManager
|
|
:param required_images: list
|
|
"""
|
|
LOG.info("Check that required containers exist")
|
|
for node_name in underlay.node_names():
|
|
self.required_images_exists(node_name, underlay, required_images)
|
|
|
|
def check_number_kube_nodes(self, underlay, k8sclient):
|
|
"""Check number of slaves"""
|
|
LOG.info("Check number of nodes")
|
|
k8s_nodes = k8sclient.nodes.list()
|
|
node_names = underlay.node_names()
|
|
assert len(k8s_nodes) == len(node_names),\
|
|
"Check number k8s nodes failed!"
|
|
|
|
def check_etcd_health(self, underlay):
|
|
node_names = underlay.node_names()
|
|
cmd = "etcdctl cluster-health | grep -c 'got healthy result'"
|
|
|
|
etcd_nodes = underlay.sudo_check_call(
|
|
cmd, node_name=node_names[0])['stdout'][0]
|
|
assert int(etcd_nodes) == len(node_names),\
|
|
"Number of etcd nodes is {0}," \
|
|
" should be {1}".format(int(etcd_nodes), len(node_names))
|
|
|
|
def create_env_snapshot(self, name, hardware, description=None):
|
|
hardware.create_snapshot(name, description=description)
|