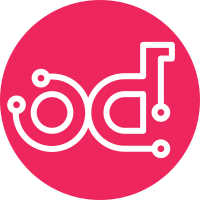
This patch introduces a keepalived manager which will be used for the blueprint blueprint l3-high-availability. The manager can create a keepalived.conf compliant configuration, start, stop and restart the service, as well as create keepalived notification scripts. Implements: blueprint l3-high-availability Change-Id: I1ba9f332778f27de950d9e97d4fb4a337f6f26da Co-Authored-By: Assaf Muller <amuller@redhat.com>
61 lines
2.3 KiB
Python
61 lines
2.3 KiB
Python
# Copyright (c) 2014 Red Hat, Inc.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from oslo.config import cfg
|
|
|
|
from neutron.agent.common import config
|
|
from neutron.agent.linux import external_process
|
|
from neutron.agent.linux import keepalived
|
|
from neutron.openstack.common import log as logging
|
|
from neutron.tests.functional import base as functional_base
|
|
from neutron.tests.unit.agent.linux import test_keepalived
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
|
|
class KeepalivedManagerTestCase(functional_base.BaseSudoTestCase,
|
|
test_keepalived.KeepalivedConfBaseMixin):
|
|
def setUp(self):
|
|
super(KeepalivedManagerTestCase, self).setUp()
|
|
self.check_sudo_enabled()
|
|
self._configure()
|
|
|
|
def _configure(self):
|
|
cfg.CONF.set_override('debug', True)
|
|
config.setup_logging(cfg.CONF)
|
|
config.register_root_helper(cfg.CONF)
|
|
cfg.CONF.set_override('root_helper', self.root_helper, group='AGENT')
|
|
|
|
def test_keepalived_spawn(self):
|
|
expected_config = self._get_config()
|
|
manager = keepalived.KeepalivedManager('router1', expected_config,
|
|
conf_path=cfg.CONF.state_path,
|
|
root_helper=self.root_helper)
|
|
self.addCleanup(manager.disable)
|
|
|
|
manager.spawn()
|
|
process = external_process.ProcessManager(
|
|
cfg.CONF,
|
|
'router1',
|
|
self.root_helper,
|
|
namespace=None,
|
|
pids_path=cfg.CONF.state_path)
|
|
self.assertTrue(process.active)
|
|
|
|
config_path = manager._get_full_config_file_path('keepalived.conf')
|
|
with open(config_path, 'r') as config_file:
|
|
config_contents = config_file.read()
|
|
self.assertEqual(expected_config.get_config_str(), config_contents)
|